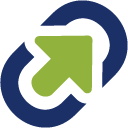
TinyUrl
URL ShortenersWorking with the Public API Docs of https://tinyurl.com/app/dev in JavaScript
Accessing public APIs is an excellent way of integrating different applications and services, giving developers freedom to create sophisticated applications. In this blog post, we'll look at how to use the public API docs of https://tinyurl.com/app/dev in JavaScript.
Getting Started
To get started, you'll need to obtain an API key from https://tinyurl.com/app/dev. This API key is required for authentication with the server, and without it, you won't be able to access any data.
Once you've obtained the API key, you can start integrating the API into your application. The first step is to authenticate with the server using your API key. Below is an example of how to authenticate with the server using the fetch()
function in JavaScript.
const apiKey = '<YOUR_API_KEY>'
const url = 'https://tinyurl.com/app/dev/authenticate'
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => {
if (response.ok) {
return response.json()
}
throw new Error('Authentication failed')
})
.then(data => {
console.log(data)
})
.catch(error => {
console.error(error)
})
Queries
Once authenticated, you can start making queries to the server for data. The API documentation at https://tinyurl.com/app/dev provides a list of available endpoints and parameters. Below is an example of how to query the server for data using the fetch()
function in JavaScript.
const apiKey = '<YOUR_API_KEY>'
const url = 'https://tinyurl.com/app/dev/query'
const query = {
endpoint: 'users',
parameters: {
page: 1,
limit: 10
}
}
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify(query)
})
.then(response => {
if (response.ok) {
return response.json()
}
throw new Error('Failed to retrieve data')
})
.then(data => {
console.log(data)
})
.catch(error => {
console.error(error)
})
Modifying Data
The API also allows modifying data on the server. To modify data, you'll need to use the PUT
or POST
methods in the fetch()
function in JavaScript. Below is an example of modifying data on the server.
const apiKey = '<YOUR_API_KEY>'
const url = 'https://tinyurl.com/app/dev/data'
const data = {
endpoint: 'users',
payload: {
id: 1,
name: 'John'
}
}
fetch(url, {
method: 'PUT', // or 'POST'
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify(data)
})
.then(response => {
if (response.ok) {
return response.json()
}
throw new Error('Failed to modify data')
})
.then(data => {
console.log(data)
})
.catch(error => {
console.error(error)
})
Conclusion
In this blog post, we've looked at how to use the public API docs of https://tinyurl.com/app/dev in JavaScript. You can now start integrating the API into your application for a seamless flow of data. Happy coding!