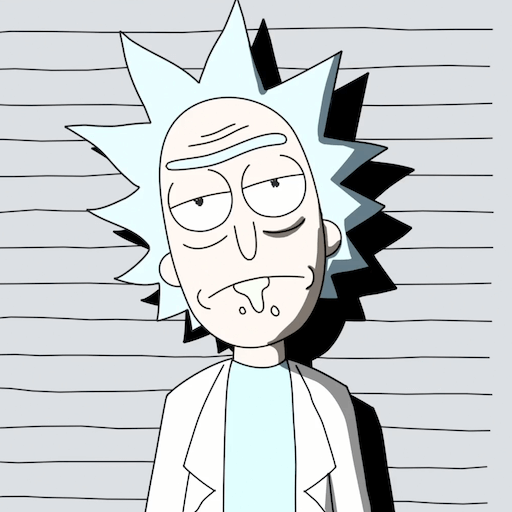
📚 Documentation & Examples
Everything you need to integrate with Rick and Morty
🚀 Quick Start Examples
// Rick and Morty API Example
const response = await fetch('https://rickandmortyapi.com', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Rick and Morty API
Are you a fan of the hit animated sci-fi sitcom, Rick and Morty? If so, you'll be excited to know that there is a public API available that provides access to a wealth of data about this bizarre and hilarious world of interdimensional travel and crazy adventures.
The Rick and Morty API (https://rickandmortyapi.com) allows developers to programmatically retrieve information about characters, locations, and episodes of the show. In this article, we'll explore how to use the API and provide example code in Javascript.
Getting Started
To start using the Rick and Morty API, you must first make sure that you have Javascript installed on your computer.
Installing Javascript
If you haven't already, you can install Node.js which will include Javascript.
Retrieving Information from the API
The Rick and Morty API has a number of endpoints that allow you to retrieve information about the show. Here are some examples:
Retrieving a Specific Character
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/character/1')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the character information for Rick Sanchez
Retrieving Multiple Characters
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/character?page=2')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the information for all characters on page 2
Retrieving a Specific Location
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/location/1')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the information for the first location, the Earth (C-137)
Retrieving Multiple Locations
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/location?page=2')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the information for all locations on page 2
Retrieving a Specific Episode
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/episode/1')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the information for the first episode, "Pilot"
Retrieving Multiple Episodes
const fetch = require('node-fetch');
fetch('https://rickandmortyapi.com/api/episode?page=2')
.then(res => res.json())
.then(json => console.log(json));
// the above code will print the information for all episodes on page 2
Conclusion
The Rick and Morty API provides developers with a fun and unique way to interact with one of the most beloved shows in recent memory. With access to a vast array of character, location, and episode data, there is plenty of room for creativity in building new applications using this API. With the code examples provided in this article, you should be well-equipped to begin exploring this incredible resource.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes