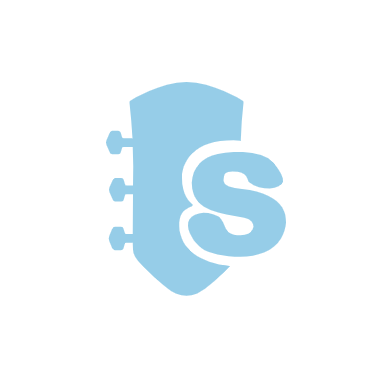
Songsterr API
MusicAccessing Songsterr's API with JavaScript
Songsterr provides a public API that allows developers to access their vast collection of guitar tabs, bass tabs, and drum tabs.
Here's an example of how to use the Songsterr API in JavaScript:
fetch('https://www.songsterr.com/a/wa/bestMatchForQueryStringPart?s=enter+sandman')
.then(response => response.json())
.then(data => console.log(data));
This example sends a request to the Songsterr API for the best match of the search query "enter sandman." The response data is returned in JSON format, which is logged to the console.
Here are some other examples of API requests:
Get a specific tab
fetch('https://www.songsterr.com/a/wa/bestMatchForQueryStringPart?s=enter+sandman')
.then(response => response.json())
.then(data => {
const id = data[0].id;
fetch(`https://www.songsterr.com/a/wa/view?r=${id}`)
.then(response => response.text())
.then(data => console.log(data));
});
This example first retrieves the ID of the best match for the search query "enter sandman," then uses that ID to fetch the full tab data in HTML format.
Get a list of tabs by artist
fetch('https://www.songsterr.com/a/ra/songs/byartists.json?artists=metallica,led+zeppelin')
.then(response => response.json())
.then(data => console.log(data));
This example retrieves a list of tabs by the artists "Metallica" and "Led Zeppelin" in JSON format.
Search for tabs by keyword
fetch('https://www.songsterr.com/a/ra/songs.json?pattern=master+of+puppets')
.then(response => response.json())
.then(data => console.log(data));
This example searches for tabs that match the keyword "master of puppets" in JSON format.
These are just a few examples of what you can do with the Songsterr API. For more information on how to use the API, refer to the documentation at https://www.songsterr.com/a/wa/api/?ref=apilist.fun. Happy coding!