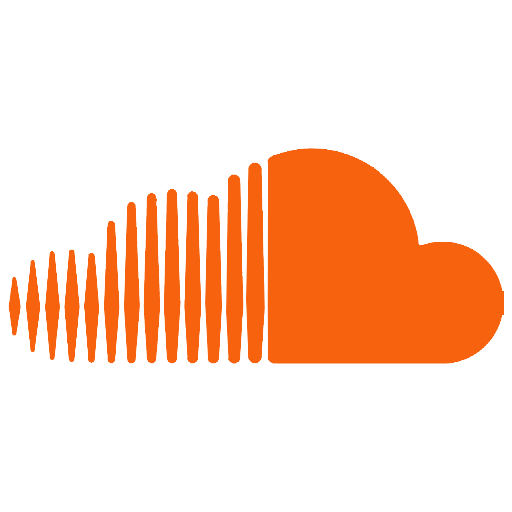
Soundcloud
MusicExploring the SoundCloud API with JavaScript
SoundCloud is a popular online music sharing platform that offers a variety of APIs to interact with its service programmatically. In this blog post, we will explore some of the most commonly used SoundCloud APIs and see how we can use them in JavaScript.
Getting Started
Before we begin, we need to create a SoundCloud account and register an app to get an API key. Head over to https://developers.soundcloud.com/ and follow the instructions for registering a new app.
Once we have the API key, we are ready to start exploring the SoundCloud API.
API Endpoints
The SoundCloud API offers a variety of endpoints to interact with the service. Some of the most commonly used API endpoints are:
/users/{user_id}
: Retrieve information about a user/users/{user_id}/tracks
: Retrieve a list of tracks uploaded by a user/tracks/{track_id}
: Retrieve information about a track/tracks/{track_id}/comments
: Retrieve a list of comments on a track/tracks/{track_id}/play
: Play a track
Example Code
Let's see some example code that uses the SoundCloud API in JavaScript. We will use the fetch
API to make HTTP requests to the SoundCloud API.
Get User Information
const API_URL = "https://api.soundcloud.com";
const USER_ID = "123"; // Replace with the user ID you want to retrieve information about
const API_KEY = "your_api_key_here"; // Replace with your SoundCloud API key
fetch(`${API_URL}/users/${USER_ID}?client_id=${API_KEY}`)
.then((response) => response.json())
.then((data) => {
console.log(data);
// Do something with user information
});
Get Tracks by User
const API_URL = "https://api.soundcloud.com";
const USER_ID = "123"; // Replace with the user ID you want to retrieve tracks from
const API_KEY = "your_api_key_here"; // Replace with your SoundCloud API key
fetch(`${API_URL}/users/${USER_ID}/tracks?client_id=${API_KEY}`)
.then((response) => response.json())
.then((data) => {
console.log(data);
// Do something with track information
});
Get Track Information
const API_URL = "https://api.soundcloud.com";
const TRACK_ID = "123"; // Replace with the track ID you want to retrieve information about
const API_KEY = "your_api_key_here"; // Replace with your SoundCloud API key
fetch(`${API_URL}/tracks/${TRACK_ID}?client_id=${API_KEY}`)
.then((response) => response.json())
.then((data) => {
console.log(data);
// Do something with track information
});
Get Comments on a Track
const API_URL = "https://api.soundcloud.com";
const TRACK_ID = "123"; // Replace with the track ID you want to retrieve comments from
const API_KEY = "your_api_key_here"; // Replace with your SoundCloud API key
fetch(`${API_URL}/tracks/${TRACK_ID}/comments?client_id=${API_KEY}`)
.then((response) => response.json())
.then((data) => {
console.log(data);
// Do something with comments
});
Play a Track
const API_URL = "https://api.soundcloud.com";
const TRACK_ID = "123"; // Replace with the track ID you want to play
const API_KEY = "your_api_key_here"; // Replace with your SoundCloud API key
const player = new Audio(`${API_URL}/tracks/${TRACK_ID}/stream?client_id=${API_KEY}`);
player.play();
Conclusion
In this blog post, we have explored some of the most commonly used SoundCloud API endpoints and seen how we can use them in JavaScript. The SoundCloud API offers many other endpoints and features that we haven't covered here, but hopefully, this post will serve as a starting point for your exploration of the SoundCloud API.