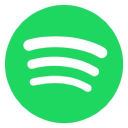
Spotify
MusicA Beginner's Guide to Spotify's Web API
Spotify's Web API is a powerful tool for developers to integrate streaming music and user data into their applications. In this tutorial, we'll go over the basics of the API and provide example code in JavaScript.
Getting Started
First, you'll need to create a Spotify developer account and register your app to obtain an API key. Once you have your API key, you can start making requests to the Web API endpoints.
Authentication
Before you can make any requests to the API, you'll need to authenticate your app. The Web API uses OAuth 2.0 for authentication, which means you'll need to obtain an access token. You can do this by making a POST request to the following endpoint:
https://accounts.spotify.com/api/token
Here's an example code snippet in JavaScript:
const client_id = 'YOUR_CLIENT_ID';
const client_secret = 'YOUR_CLIENT_SECRET';
let access_token;
async function getAccessToken() {
const response = await fetch('https://accounts.spotify.com/api/token', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
'Authorization': 'Basic ' + btoa(client_id + ':' + client_secret)
},
body: 'grant_type=client_credentials'
});
const data = await response.json();
access_token = data.access_token;
}
getAccessToken();
This code will obtain an access token using your client ID and secret, and store it in the access_token
variable.
Common API Requests
Here are some common API requests you might make using the Web API.
Search for Tracks
You can search for tracks using the following endpoint:
https://api.spotify.com/v1/search?type=track&q=QUERY
Here's an example code snippet in JavaScript:
async function searchTracks(query) {
const response = await fetch(`https://api.spotify.com/v1/search?type=track&q=${query}`, {
headers: {
'Authorization': 'Bearer ' + access_token
}
});
const data = await response.json();
return data.tracks.items;
}
This code will search for tracks matching the query
parameter and return the results as an array of objects.
Get a Track's Audio Features
You can get a track's audio features using the following endpoint:
https://api.spotify.com/v1/audio-features/ID
Here's an example code snippet in JavaScript:
async function getTrackFeatures(id) {
const response = await fetch(`https://api.spotify.com/v1/audio-features/${id}`, {
headers: {
'Authorization': 'Bearer ' + access_token
}
});
const data = await response.json();
return data;
}
This code will get the audio features of the track with the specified id
.
Get a User's Top Tracks
You can get a user's top tracks using the following endpoint:
https://api.spotify.com/v1/me/top/tracks
Here's an example code snippet in JavaScript:
async function getTopTracks() {
const response = await fetch('https://api.spotify.com/v1/me/top/tracks', {
headers: {
'Authorization': 'Bearer ' + access_token
}
});
const data = await response.json();
return data.items;
}
This code will get the current user's top tracks and return the results as an array of objects.
Conclusion
These are just a few examples of what you can do with Spotify's Web API. By using this guide and the Spotify Web API documentation, you can build your own music-focused applications and take advantage of all the amazing features Spotify has to offer.