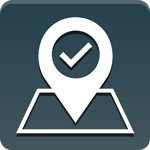
streetlayer
Data ValidationUsing the StreetLayer API in JavaScript
StreetLayer is an API that provides various geolocation data related to addresses around the world. With StreetLayer, you can quickly and easily retrieve location data for any address, including latitude, longitude, city, state, and country information.
In this blog post, we'll explore how to use the StreetLayer API in JavaScript using example code. We'll cover the basic steps of registering for an API key, making API requests, and parsing API responses.
Registering for an API Key
Before you can start using the StreetLayer API, you need to register for an API key. Follow these steps to get your own API key:
- Go to the StreetLayer website and click the Get Free API Key button.
- Fill in the registration form with your details and click Register.
- You will be redirected to the StreetLayer dashboard, where you can find your API key.
Make sure to keep your API key safe and secure.
Making API Requests
Once you have your API key, you can make API requests to retrieve geolocation information for addresses. Here's an example of how to make an API request using JavaScript:
const apiKey = "YOUR_API_KEY";
const address = "1600+Amphitheatre+Parkway,+Mountain+View,+CA";
const url = `https://api.streetlayer.com/v2/validate?access_key=${apiKey}&address=${address}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In this example, we're using the fetch
function to send an HTTP GET request to the StreetLayer API. We're passing our API key and the address we want to retrieve information for as URL parameters.
The API will respond with a JSON object containing the geolocation information for the address.
Parsing API Responses
Once we've received a response from the API, we can parse the JSON object to extract the information we need. Here's an example of how to do this:
const apiKey = "YOUR_API_KEY";
const address = "1600+Amphitheatre+Parkway,+Mountain+View,+CA";
const url = `https://api.streetlayer.com/v2/validate?access_key=${apiKey}&address=${address}`;
fetch(url)
.then(response => response.json())
.then(data => {
const latitude = data.data.latitude;
const longitude = data.data.longitude;
const city = data.data.city;
const state = data.data.state;
const country = data.data.country;
console.log(`Latitude: ${latitude}`);
console.log(`Longitude: ${longitude}`);
console.log(`City: ${city}`);
console.log(`State: ${state}`);
console.log(`Country: ${country}`);
})
.catch(error => {
console.error(error);
});
In this example, we're extracting the latitude, longitude, city, state, and country information from the data
object that's returned by the API. We're then logging this information to the console.
Conclusion
In this blog post, we covered the basic steps of using the StreetLayer API in JavaScript. We showed how to register for an API key, make API requests, and parse API responses.
The StreetLayer API offers a powerful way to retrieve geolocation information for addresses around the world. By following the example code provided here, you should be able to start using the API in your own JavaScript projects.