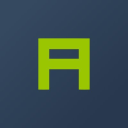
Aylien Text Analysis
Text AnalysisExploring Aylien's Public API Docs
Aylien's Public API Docs is a must-visit website for developers who want to build their own natural language processing applications. The website offers useful APIs that enable developers to extract meaningful insights from text-based content.
In this article, we will explore some of Aylien's APIs and provide code examples in JavaScript.
Sentiment Analysis API
The Sentiment Analysis API can be used to analyze the overall sentiment of a piece of text. The API outputs a polarity score ranging from -1 to 1, with negative values indicating negative sentiment and positive values indicating positive sentiment.
Requesting an API Key
Before we can use the Sentiment Analysis API, we need to obtain an API key from Aylien. You can obtain an API key by signing up here.
Code Example
const AylienAPI = require('aylien_textapi');
const textapi = new AylienAPI({
application_id: '<your_application_id>',
application_key: '<your_application_key>'
});
textapi.sentiment({
text: 'I am very happy today'
}, function(error, response) {
if (error === null) {
console.log(response);
}
});
Entity Extraction API
The Entity Extraction API can be used to extract entities from text, such as people, organizations, and locations. The API returns a JSON object containing the extracted entities.
Code Example
const AylienAPI = require('aylien_textapi');
const textapi = new AylienAPI({
application_id: '<your_application_id>',
application_key: '<your_application_key>'
});
textapi.entities({
text: 'John Smith is a developer at Google in Mountain View, California'
}, function(error, response) {
if (error === null) {
console.log(response.entities);
}
});
Concept Extraction API
The Concept Extraction API can be used to extract concepts from text, such as ideas and abstract concepts. The API returns a JSON object containing the extracted concepts.
Code Example
const AylienAPI = require('aylien_textapi');
const textapi = new AylienAPI({
application_id: '<your_application_id>',
application_key: '<your_application_key>'
});
textapi.concepts({
text: 'The painting depicts a woman staring out of a window'
}, function(error, response) {
if (error === null) {
console.log(response.concepts);
}
});
Conclusion
Aylien's Public API Docs is a valuable resource for developers who want to incorporate natural language processing into their applications. With the examples provided in this article, you can start using some of Aylien's APIs in your JavaScript projects.