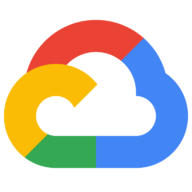
Google Cloud Natural
Text AnalysisGoogle Cloud Natural Language API Example Code in JavaScript
The Google Cloud Natural Language API is a Public API that allows you to extract insights from text using Machine Learning models developed by Google. It can analyze text and return information about the sentiment, entities, and syntax of the text.
In this blog post, we will explore how to use the Google Cloud Natural Language API in JavaScript and provide example code snippets.
Requirements
Before we start looking at the code snippets, you need to have the following:
- A Google Cloud account
- Access to the Google Cloud Console
- A project in the Google Cloud Console with the Cloud Natural Language API enabled
Getting Started
To use the Google Cloud Natural Language API in JavaScript, you need to follow these steps:
-
Install the
@google-cloud/language
package using npm:npm install @google-cloud/language
-
Authenticate with Google Cloud using a service account and the
GOOGLE_APPLICATION_CREDENTIALS
environment variable. You can create a new service account in the Google Cloud Console and download its JSON key file. Then, set theGOOGLE_APPLICATION_CREDENTIALS
environment variable to point to the path of the JSON key file. For example:export GOOGLE_APPLICATION_CREDENTIALS="/path/to/key.json"
-
Write the code to use the API.
Example Code Snippets
Analyze Sentiment
Analyze the sentiment of a piece of text:
const {LanguageServiceClient} = require('@google-cloud/language');
const client = new LanguageServiceClient();
async function analyzeSentiment() {
const document = {
content: 'I am so happy and grateful to be alive.',
type: 'PLAIN_TEXT',
};
const [result] = await client.analyzeSentiment({document});
const sentiment = result.documentSentiment;
console.log(`Score: ${sentiment.score}`);
console.log(`Magnitude: ${sentiment.magnitude}`);
}
analyzeSentiment();
Analyze Entities
Extract entities (e.g. people, places, organizations) from a piece of text:
async function analyzeEntities() {
const document = {
content: 'Sundar Pichai is the CEO of Google.',
type: 'PLAIN_TEXT',
};
const [result] = await client.analyzeEntities({document});
const entities = result.entities;
console.log('Entities:');
entities.forEach(entity => {
console.log(entity.name);
console.log(` - Type: ${entity.type}`);
console.log(` - Salience: ${entity.salience}`);
});
}
analyzeEntities();
Analyze Syntax
Extract the syntax (e.g. parts of speech, dependency labels) from a piece of text:
async function analyzeSyntax() {
const document = {
content: 'I am learning how to use the Google Cloud Natural Language API.',
type: 'PLAIN_TEXT',
};
const [result] = await client.analyzeSyntax({document});
const tokens = result.tokens;
console.log('Tokens:');
tokens.forEach(token => {
console.log(token.text.content);
console.log(` - Part of Speech: ${token.partOfSpeech.tag}`);
console.log(` - Lemma: ${token.lemma}`);
});
}
analyzeSyntax();
Conclusion
In this blog post, we explored how to use the Google Cloud Natural Language API in JavaScript and provided example code snippets for analyzing sentiment, entities, and syntax in text. The Google Cloud Natural Language API is a powerful tool that can help you extract valuable insights from text and improve the accuracy of your applications.