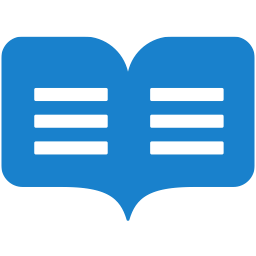
Semantira
Text AnalysisIntroduction
Are you looking for a powerful and easy-to-use natural language processing API? Look no further than Semantria! With Semantria, you can extract valuable insights from your text data with just a few lines of code. In this blog, we'll take a look at Semantria's public API documentation and provide example code in JavaScript.
Getting Started
Before we dive into the code, let's take a quick look at Semantria's API documentation. The documentation can be found at https://semantria.readme.io/docs. Here, you can find all the information you'll need to get started with the Semantria API. You can also get an API key by creating an account on the Semantria website.
Example Code
Now, let's take a look at some example code. In this example, we'll be using the Semantria Node.js package to make requests to the Semantria API.
const Semantria = require('semantria');
const semantria = new Semantria({
consumerKey: 'YOUR_CONSUMER_KEY',
consumerSecret: 'YOUR_CONSUMER_SECRET',
useCompression: true
});
// Example sentiment analysis request
semantria.createDocument(
{
id: '1',
text: 'I love this product!',
language: 'English'
},
(err, res) => {
if (err) {
console.error(err);
return;
}
console.log(res);
}
);
// Example entity extraction request
semantria.createBatchDocuments(
[
{
id: '1',
text: 'I love Nike shoes and Adidas clothes',
language: 'English'
}
],
{
language: 'English',
documentType: 'text',
processingMode: 'disambiguation',
entityExtraction: {
enabled: true,
selectionPolicy: 'strict',
entityThreshold: 0.05
}
},
(err, res) => {
if (err) {
console.error(err);
return;
}
console.log(res);
}
);
In this example, we're using createDocument
and createBatchDocuments
methods to analyze sentiment and extract entities, respectively. These methods take a document or an array of documents as the first argument and a configuration object as the second argument. The configuration object contains parameters such as the language of the text, the type of document (text or HTML), and whether entity extraction should be enabled.
Conclusion
That's it for our brief introduction to Semantria's public API documentation and example code in JavaScript. With Semantria's powerful API, you can extract valuable insights from your text data with ease. Whether you're analyzing sentiment, extracting entities, or performing any other natural language processing task, Semantria has you covered.