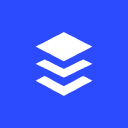
Buffer
SocialPublic API Docs for Buffer
If you're looking to integrate your app with Buffer's social media management platform, you're in the right place. The Buffer API provides developers with a wide range of functionality to help them build powerful social media management tools.
Getting Started
To get started with the Buffer API, you'll first need to authenticate your app with Buffer. You can do this by creating a Buffer account and generating an access token. Once you have your access token, you can use it to make requests to the Buffer API.
JavaScript Example Code
Here are some example requests you can make to the Buffer API using JavaScript:
Get Profile Information
This example code retrieves profile information for a user in Buffer:
const BufferAPI = require('buffer')
const buffer = new BufferAPI({
accessToken: 'YOUR_ACCESS_TOKEN',
})
buffer.get('/profiles?service=twitter').then((data) => {
console.log(data)
})
Get Pending Updates
This example code retrieves a list of pending updates in a user's Buffer account:
const BufferAPI = require('buffer')
const buffer = new BufferAPI({
accessToken: 'YOUR_ACCESS_TOKEN',
})
buffer.get('/profiles/ID/updates/pending').then((data) => {
console.log(data)
})
Create a New Update
This example code creates a new update in a user's Buffer account:
const BufferAPI = require('buffer')
const buffer = new BufferAPI({
accessToken: 'YOUR_ACCESS_TOKEN',
})
buffer.post('/updates/create', {
profile_ids: ['PROFILE_ID'],
text: 'This is a test update from my app',
}).then((data) => {
console.log(data)
})
Delete an Update
This example code deletes an existing update from a user's Buffer account:
const BufferAPI = require('buffer')
const buffer = new BufferAPI({
accessToken: 'YOUR_ACCESS_TOKEN',
})
buffer.delete('/updates/ID').then(() => {
console.log('Update deleted successfully!')
})
These are just a few examples of what you can do with the Buffer API. Be sure to check out the full documentation for more information on all the available API endpoints and parameters. Happy coding!