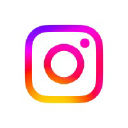
The Instagram API provides developers with powerful tools for integrating Instagram's features into their applications. With functionalities such as Instagram Login, users can easily authenticate and connect their accounts, enhancing the user experience while ensuring secure access. The API also allows seamless sharing of content on Instagram, enabling applications to post photos and videos directly to a user's feed. Leveraging social plugins grants developers the ability to embed Instagram content on their websites, creating an interactive and engaging environment for users. This functionality not only boosts interaction but also increases the visibility of Instagram content, driving more traffic and enhancing overall engagement.
Using the Instagram API can lead to several benefits, including:
- Simplified user authentication through Instagram Login.
- Enhanced user engagement with easy content sharing features.
- Increased visibility of products and services on social media platforms.
- Access to rich media content, enabling diverse and engaging user experiences.
- Support for integration with apps, enhancing overall functionality and user experience.
Here’s an example of how to use the Instagram API with JavaScript:
const clientId = 'YOUR_CLIENT_ID';
const redirectUri = 'YOUR_REDIRECT_URI';
const authorizationUrl = `https://api.instagram.com/oauth/authorize?client_id=${clientId}&redirect_uri=${redirectUri}&response_type=token`;
// Redirect user for Instagram login
window.location.href = authorizationUrl;
// Example of sharing content to Instagram
function shareToInstagram(imageUrl) {
const shareUrl = `https://api.instagram.com/v1/media/share?image_url=${encodeURIComponent(imageUrl)}&access_token=YOUR_ACCESS_TOKEN`;
fetch(shareUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => {
console.log('Successfully shared to Instagram!', data);
})
.catch(error => {
console.error('Error sharing to Instagram:', error);
});
}