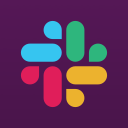
Slack
SocialThe Team Instant Messaging API from Slack is designed to enable seamless communication within teams, fostering collaboration and productivity in the workplace. This powerful API allows developers to integrate Slack’s rich messaging functionality into their applications, creating a more unified user experience. With features like sending messages, managing channels, and retrieving user information, the Slack API supports a diverse range of use cases, from automating team notifications to developing custom chatbots that enhance team interactions. The comprehensive documentation available at Slack API Documentation provides all the necessary resources to effectively implement and leverage this API for various communication needs.
Using the Slack Team Instant Messaging API offers numerous benefits, including enhancing team engagement, increasing efficiency by automating tasks, and allowing for better organization of conversations through channels. Additionally, it enables real-time messaging, which keeps teams connected no matter where they are. By incorporating the Slack API into your applications, you can transform the way your team communicates and collaborates.
- Improved team collaboration and engagement
- Real-time messaging capabilities
- Automation of routine tasks and notifications
- Enhanced organization of discussions through channels
- Integration with other tools and services for a streamlined workflow
Here’s a simple JavaScript code example to send a message using the Slack API:
const axios = require('axios');
const sendMessageToSlack = async (channel, text) => {
const token = 'YOUR_SLACK_API_TOKEN'; // Replace with your Slack API token
const url = 'https://slack.com/api/chat.postMessage';
try {
const response = await axios.post(url, {
channel: channel,
text: text
}, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
if (response.data.ok) {
console.log('Message sent successfully:', response.data);
} else {
console.error('Error sending message:', response.data.error);
}
} catch (error) {
console.error('Error:', error);
}
};
// Usage
sendMessageToSlack('#general', 'Hello, team!'); // Sends a message to the #general channel