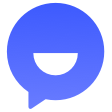
TamTam
SocialThe TamTam Bot API provides a robust solution for developers looking to create and manage bots that interact seamlessly within the TamTam messaging platform. With comprehensive documentation available at dev.tamtam.chat, this API empowers users to automate tasks, engage with their audience, and enhance the overall user experience. By leveraging the features offered by this API, developers can build interactive bots that perform various functions such as sending messages, responding to user inquiries, and managing group interactions. The easy-to-understand structure of the API allows for rapid development, making it an invaluable tool for businesses and individual developers alike.
Using the TamTam Bot API offers numerous benefits, including:
- Streamlined integration with the TamTam messaging platform for immediate reach to users.
- Enhanced engagement through personalized bot interactions tailored to individual preferences.
- Automation of repetitive tasks and responses, saving time and resources.
- Flexibility to build complex bots with varied functionalities such as polls, games, and information retrieval.
- Comprehensive support and resources from the TamTam developer community, ensuring assistance and guidance when needed.
Here’s a simple JavaScript code example for calling the TamTam Bot API to send a message:
const axios = require('axios');
const sendMessage = async (userId, message) => {
const token = 'YOUR_BOT_TOKEN';
const url = `https://api.tamtam.chat/messages/send`;
const data = {
peer_id: userId,
text: message
};
try {
const response = await axios.post(url, data, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Message sent successfully:', response.data);
} catch (error) {
console.error('Error sending message:', error);
}
};
// Example usage
sendMessage('USER_PEER_ID', 'Hello from my TamTam bot!');