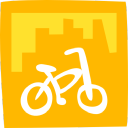
City Bikes API
Sports & FitnessExploring the Public API of CityBik.es
If you're looking to build a bike-sharing app or website, you might want to take a look at CityBik.es. This platform provides data and information about more than 1,600 bicycle-sharing systems around the world, including real-time data about bike availability, location, and usage.
The CityBik.es API is open and free to use, with no authentication or API key required. You can use it to retrieve information about the bike-sharing systems and stations, as well as real-time updates about the status of the bikes.
API Endpoints
The CityBik.es API has several endpoints that allow you to access different kinds of data. Here are some of the main ones:
-
/networks
: This endpoint returns information about all the bike-sharing networks available in the CityBik.es platform. You can use this endpoint to retrieve network identifiers, names, and locations. -
/networks/{network_id}
: This endpoint returns information about a specific network, including the stations and the status of the bikes. You need to replace{network_id}
with the network identifier you want to retrieve information about. -
/networks/{network_id}/stations
: This endpoint returns information about the stations of a specific network, including the location, available bikes, and free slots. You need to replace{network_id}
with the network identifier you want to retrieve information about. -
/networks/{network_id}/stations/{station_id}
: This endpoint returns information about a specific station of a specific network, including the location, available bikes, and free slots. You need to replace{network_id}
with the network identifier and{station_id}
with the station identifier you want to retrieve information about.
Using the API in JavaScript
Here's an example of how you can use the CityBik.es API in JavaScript to retrieve information about all the bike-sharing networks available in the platform:
const apiUrl = 'http://api.citybik.es/v2/networks';
fetch(apiUrl)
.then((response) => response.json())
.then((data) => {
// Do something with the data
console.log(data);
})
.catch((error) => {
// Handle errors
console.error(error);
});
This code makes an HTTP request to the /networks
endpoint using the fetch
API. The fetch
function returns a promise that resolves to the response from the server, which we then convert to JSON using the json
method.
Once we have the data, we can do whatever we want with it. In this case, we simply log it to the console.
Here's another example of how we can use the CityBik.es API in JavaScript to retrieve information about a specific network and its stations:
const networkId = 'visa-frankfurt';
const apiUrl = `http://api.citybik.es/v2/networks/${networkId}/stations`;
fetch(apiUrl)
.then((response) => response.json())
.then((data) => {
// Do something with the data
console.log(data);
})
.catch((error) => {
// Handle errors
console.error(error);
});
In this case, we're using string interpolation to build the URL for the request, replacing the {network_id}
placeholder with the actual identifier of the network we want to retrieve information about.
We then make an HTTP request to the /networks/{network_id}/stations
endpoint and handle the response in the same way as in the previous example.
Conclusion
The CityBik.es API provides a wealth of data and information about bike-sharing networks around the world, which can be very useful if you're working on a bike-sharing app or website.
Using the API in JavaScript is straightforward, thanks to the fetch
API and the flexibility of the platform. With a few lines of code, you can retrieve information about the networks, the stations, and the status of the bikes, and use it to build engaging and useful applications.