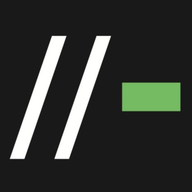
Football-Data.org
Sports & FitnessFootball Data. The dev-friendly football API RESTful. Reliable. Free to use. Easy to integrate. football-data.org provides football data and statistics (live scores, fixtures, tables, squads, lineups/subs, etc.) in a machine-readable way. Our free (RESTful) API in JSON representation is used by thousands of developers to power websites and mobile apps with football data. Access to the top football competitions is and will be free forever as this was the initial purpose to setup the project. However if you need more competitions (or in-depth data), we have several paid plans available to serve your needs. The exhaustive documentation, code samples and libraries will help you get up and running quickly.
π Documentation & Examples
Everything you need to integrate with Football-Data.org
π Quick Start Examples
// Football-Data.org API Example
const response = await fetch('http://api.football-data.org/index', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the football-data.org API in JavaScript
If you're a football enthusiast and are looking for a reliable API to get football-related data, then the football-data.org API is definitely worth exploring. This API provides access to various football-related data such as league standings, fixtures, players and more.
In this article, we will explore how to use the football-data.org API in JavaScript. We will also look at a few examples that demonstrate how to consume data from the API.
Getting started
To get started with the football-data.org API, you will need to create an account and request an API token. Once you have your token, you can start using the API by sending HTTP requests to the API endpoints.
Making API requests
To make HTTP requests to the football-data.org API, we can use various HTTP libraries available in JavaScript such as Axios or the built-in Fetch API.
Here is an example using Axios:
import axios from 'axios';
const API_TOKEN = 'YOUR_API_TOKEN';
const baseUrl = 'http://api.football-data.org/v2';
/**
* Example function to get league standings.
*/
async function getLeagueStandings(leagueId) {
const url = `${baseUrl}/competitions/${leagueId}/standings`;
try {
const response = await axios.get(url, {
headers: {
'X-Auth-Token': API_TOKEN
}
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
// Call the function with the league ID
getLeagueStandings(2021); // Premier League
In this example, we are making a GET request to the standings
endpoint of the Premier League using Axios. We are passing our API token in the headers of the request. Once the request is successful, the data is logged to the console.
Example API calls
Here are a few more examples of how to make API requests to the football-data.org API using JavaScript.
Get all competitions
async function getAllCompetitions() {
const url = `${baseUrl}/competitions`;
try {
const response = await axios.get(url, {
headers: {
'X-Auth-Token': API_TOKEN
}
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
getAllCompetitions();
In this example, we are making a GET request to the competitions
endpoint to get all available competitions.
Get the details of a single competition
async function getCompetitionDetails(competitionId) {
const url = `${baseUrl}/competitions/${competitionId}`;
try {
const response = await axios.get(url, {
headers: {
'X-Auth-Token': API_TOKEN
}
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
getCompetitionDetails(2021); // Premier League
In this example, we are making a GET request to the competitions
endpoint to get the details of a single competition, which in our case is the Premier League.
Get fixtures of a specific team
async function getTeamFixtures(teamId) {
const url = `${baseUrl}/teams/${teamId}/matches`;
try {
const response = await axios.get(url, {
headers: {
'X-Auth-Token': API_TOKEN
}
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
getTeamFixtures(57); // Arsenal FC
In this example, we are making a GET request to the teams
endpoint to get all fixtures of a specific team, which in our case is Arsenal FC.
Conclusion
As we have seen, the football-data.org API is a powerful and useful tool for getting football-related data. Using JavaScript, we can easily consume the API by making HTTP requests to its various endpoints.
We have explored a few examples of using the API, but there are many more endpoints available to explore. Be sure to check out the API documentation for more information.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes