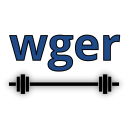
wger API
Sports & FitnessExploring the Wger API with JavaScript
Are you looking for a reliable fitness API to integrate into your project? Look no further because Wger API is the one. Here is a quick guide on how to use Wger API with JavaScript.
Getting started
Firstly, you need to create an account and have an API key. Once you have an API key, you can start making requests to the API endpoint.
Making a request
To make a request to Wger API, you need to use the fetch
method which is a web API for making API requests in JavaScript.
Here is an example code in JavaScript to get all exercises in Wger API.
fetch(" https://wger.de/api/v2/exercise/")
.then((response) => response.json())
.then((data) => console.log(data));
The above code will return a response that contains all the exercises in Wger API.
Authenticating request
If you want to make a request that requires user authentication, you need to pass your API key in the request header. Here is an example code in JavaScript to authenticate a request to get all user's logs:
fetch("https://wger.de/api/v2/workoutlog/", {
headers: {
"Authorization": "Token " + api_key,
"Content-Type": "application/json"
}
})
.then((response) => response.json())
.then((data) => console.log(data));
Make sure to replace api_key
with your actual API key.
Filtering data
Wger API allows you to filter data by specific parameters. For example, if you want to get all exercises that belong to a specific category, you can add a category
parameter to the URL.
Here is an example code in JavaScript to get all exercises that belong to the category with ID 10
:
fetch("https://wger.de/api/v2/exercise/?category=10")
.then((response) => response.json())
.then((data) => console.log(data));
Conclusion
The Wger API is a powerful and easy-to-use API that is free to use. With the above examples, you can get started with using Wger API in your next project.