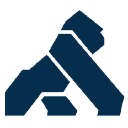
mashape
Sports & Fitnesslive-score. In 2011 we launched the first API Marketplace, which has grown to over 15,000 APIs and 200,000 developers in the community. Since then we’ve built a lot of useful tools for ourself. Last April we decided to spin off several products and make them available to everyone. Starting with open-sourcing Mockbin, Kong and HTTPsnippet, we most recently released Galileo for API Analytics. Late last year we acquired Gelato.io – our family is now bigger than ever and it was the right time to update our main entrance door. Kong is trusted by over 200 enterprises worldwide, from startups to Fortune 500 companies.
📚 Documentation & Examples
Everything you need to integrate with mashape
🚀 Quick Start Examples
// mashape API Example
const response = await fetch('https://konghq.com/blog/mashape-has-a-new-homepage/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introducing Mashape - A Public API Platform
Mashape provides a one-stop solution for developers to rapidly prototype, test, and deploy APIs. With Mashape, there's no need to worry about hosting, scaling or monitoring your APIs. Instead, you can focus on building the features that matter most to your users.
Mashape API Examples in JavaScript
Mashape offers a variety of APIs for developers to integrate into their projects. Here are some examples of how to use Mashape APIs with JavaScript:
Example 1: Sentiment Analysis API
The Sentiment Analysis API allows you to analyze the sentiment of text. Here's some sample code in JavaScript:
const unirest = require("unirest");
const req = unirest("POST", "https://aylien-text.p.rapidapi.com/sentiment");
req.query({
"text": "I love Mashape!"
});
req.headers({
"x-rapidapi-host": "aylien-text.p.rapidapi.com",
"x-rapidapi-key": "YOUR-API-KEY-HERE",
"content-type": "application/x-www-form-urlencoded"
});
req.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.body);
});
Example 2: Twilio API
Twilio allows you to send SMS messages and place phone calls. Here's some sample code in JavaScript:
const accountSid = 'YOUR-TWILIO-ACCOUNT-SID';
const authToken = 'YOUR-TWILIO-AUTH-TOKEN';
const client = require('twilio')(accountSid, authToken);
client.messages
.create({
body: 'Hello from Mashape!',
from: '+TWILIO-PHONE-NUMBER',
to: '+RECIPIENT-PHONE-NUMBER'
})
.then(message => console.log(message.sid))
.done();
Example 3: OpenWeatherMap API
OpenWeatherMap provides weather data for any location. Here's some sample code in JavaScript:
const request = require('request');
const apiKey = 'YOUR-API-KEY-HERE';
const city = 'london';
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`;
request(url, function (err, response, body) {
if(err){
console.log('error:', error);
} else {
const weather = JSON.parse(body);
console.log(`It's currently ${weather.main.temp} degrees in ${weather.name}!`);
}
});
These are just a few examples of the broad range of APIs available on Mashape. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes