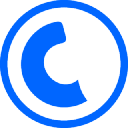
Cutt.ly
URL ShortenersThe URL shortener service offered by Cutt.ly provides an efficient way to condense lengthy URLs into concise, shareable links. This API is ideal for developers looking to enhance user experience on their platforms by simplifying link sharing. With robust features such as link management, analytics, and customization options, the Cutt.ly API allows users to shorten URLs effortlessly while tracking their performance in real-time. Whether for social media, email campaigns, or web applications, integrating this service can streamline content sharing and improve click-through rates.
Using the Cutt.ly API comes with several benefits, including:
- Easy integration with various programming languages
- Customizable short links to align with branding
- Comprehensive tracking and analytics for user engagement
- High reliability and speed for quick URL shortening
- Secure link management with an option for password protection
Here’s a JavaScript code example demonstrating how to use the Cutt.ly API to shorten a URL:
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const LONG_URL = 'https://www.example.com/some/very/long/url';
axios.post('https://cutt.ly/api/api.php', null, {
params: {
key: API_KEY,
short: LONG_URL
}
})
.then(response => {
if (response.data.url.shortLink) {
console.log('Shortened URL:', response.data.url.shortLink);
} else {
console.error('Error shortening URL:', response.data);
}
})
.catch(error => {
console.error('Error occurred:', error);
});