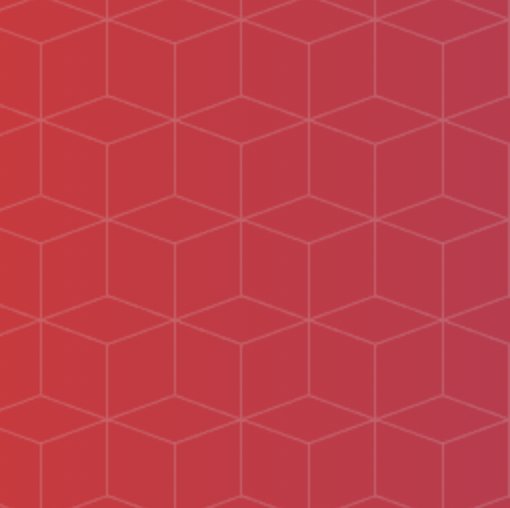
GooLNK
URL ShortenersShorten a long URL. POST request to: /api/v1/shorten Long URLs should be URL-encoded. You can not include a longUrl in the request that has &, ?, #, or other reserved parameters without first encoding it. Long URLs should not contain spaces: any longUrl with spaces will be rejected. All spaces should be either percent encoded %20 or plus encoded +. Note that tabs, newlines and trailing spaces are all indications of errors. Please remember to strip leading and trailing whitespace from any user input before shortening.
📚 Documentation & Examples
Everything you need to integrate with GooLNK
🚀 Quick Start Examples
// GooLNK API Example
const response = await fetch('https://goolnk.com/docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Goolnk Public API
Goolnk is a URL shortening service that you can use to shorten long, complicated URLs into short, shareable links. The service also provides a public API that you can use to automate the process of generating short links. In this article, we will take a closer look at the Goolnk public API, and explore how to use it with JavaScript.
Getting Started with the Goolnk API
Before we dive into some examples of how to use the Goolnk API with JavaScript, we first need to obtain an API key. You can create an account on the Goolnk website, and then generate an API key from your account dashboard.
Once you have an API key, you can start making requests to the Goolnk API. The API is accessible at the following endpoint:
https://api.goolnk.com/v1/links
Generating Short Links with the Goolnk API and JavaScript
To generate a short link with the Goolnk API, we need to send a POST request to the API endpoint, along with the long URL that we want to shorten. Here's an example JavaScript code snippet that demonstrates how to generate a short link with the Goolnk API:
const apiKey = 'your_api_key';
const longUrl = 'https://some-long-url.com';
fetch('https://api.goolnk.com/v1/links', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify({
url: longUrl
})
})
.then(response => response.json())
.then(data => console.log(`Short link: ${data.short_link}`))
.catch(error => console.error(error));
In this example, we first define our Goolnk API key and the long URL that we want to shorten. We then send a POST request to the API endpoint, along with the necessary headers and the long URL in the request body.
Once we receive a response from the API, we parse the JSON data and log the short link to the console.
Conclusion
Using the Goolnk public API with JavaScript is relatively straightforward. By sending a POST request to the API endpoint, along with the necessary headers and the long URL, we can generate short links programmatically. This can be useful if you need to generate a large number of short links or want to automate the process of generating short links for your website or application.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes