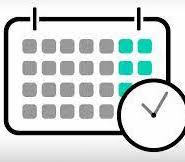
Digi Dates
CalendarDigiDates is a powerful API that provides time and date calculations for a variety of use cases. Whether you need to calculate time differences, convert time zones, or determine the duration between two dates, DigiDates.de can help. Their API is designed to be easy to integrate into your application, with comprehensive documentation and support available to ensure a smooth integration process. With DigiDates.de, you can take advantage of advanced features like business day calculations and holiday schedules, making it easier to manage complex time-related operations. So why not explore the possibilities and see how DigiDates.de can help streamline your time and date calculations today?
π Documentation & Examples
Everything you need to integrate with Digi Dates
π Quick Start Examples
// Digi Dates API Example
const response = await fetch('https://digidates.de/en/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to Digidates Public API
If you want to access data about important dates, anniversaries, and holidays, then the Digidates Public API could be just what you need. This API gives you access to a wide range of useful information about events from all over the world.
To get started with the Digidates API, you first need to sign up for an API key. You can do this by visiting https://digidates.de/en/ and following the instructions on the website. Once you have an API key, you can start making requests to the API using a variety of programming languages.
In this blog post, we'll provide some example code in JavaScript that demonstrates how to connect to the Digidates API and retrieve information about upcoming events.
Connecting to the API
To start working with the Digidates API, you'll need to make an HTTP request to the API endpoint. You can do this using the built-in fetch
function in JavaScript. Here's an example of how to connect to the API and retrieve some data:
const apiKey = 'your-api-key';
const apiUrl = `http://api.digidates.de/api/v1/?apikey=${apiKey}&command=get_events&type=holiday`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're using the fetch
function to make a GET request to the Digidates API. We're passing in our API key as a query parameter in the URL, along with the command
parameter (which tells the API what we want to do) and the type
parameter (which specifies the type of event we're interested in).
When we receive a response from the API, we're using the json
method to parse the response into a JavaScript object. We're then logging the data to the console.
Working with the API response
Once we've connected to the Digidates API and retrieved some data, we can start working with the response. The response from the API will be in JSON format, which makes it easy to parse and use.
Here's an example of how to loop through the data returned by the API and display some information about each event:
const apiKey = 'your-api-key';
const apiUrl = `http://api.digidates.de/api/v1/?apikey=${apiKey}&command=get_events&type=holiday`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
data.events.forEach(event => {
console.log(`${event.title} is on ${event.date}`);
});
})
.catch(error => console.error(error));
In this example, we're using the forEach
method to loop through the events
array in the response from the API. For each event, we're logging the title and date to the console. You could easily modify this code to display the information on a web page instead.
Conclusion
In this blog post, we've provided some example code in JavaScript that demonstrates how to connect to the Digidates Public API and retrieve information about upcoming events. This is just the tip of the iceberg - the Digidates API provides a wealth of information that you can use to build all sorts of applications.
We hope this post has been helpful in getting you started with the Digidates API. If you have any questions or comments, please feel free to leave them below. Happy coding!
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes