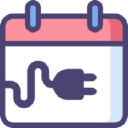
Holidays
CalendarExploring the Holiday API with JavaScript
The Holiday API provides developers with easy access to holiday data for over 230 countries with JSON responses. In this tutorial, we will use JavaScript to query the API and retrieve data for a specific country.
Setting Up
Before we begin, we need to set up our development environment. We will start by creating an HTML page with a button that calls the API when clicked.
<!DOCTYPE html>
<html>
<head>
<title>Holiday API</title>
</head>
<body>
<button onclick="getHolidays()">Get Holidays</button>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="app.js"></script>
</body>
</html>
We have also included the jQuery library and a separate JavaScript file called app.js
. We will use app.js
to make our API call.
Making the API call
The Holiday API has a simple URL structure. We need to provide a few parameters to specify the country, year and month for which we want to retrieve the holidays. We will use the fetch()
method to make the API call.
async function getHolidays() {
const url = "https://holidayapi.com/v1/holidays?key=YOUR_API_KEY&country=US&year=2022&month=1";
const response = await fetch(url);
const data = await response.json();
console.log(data);
}
In this example, we have hardcoded the parameters for the United States in January 2022. We have used YOUR_API_KEY
as a placeholder for your unique API key, which you can obtain from the Holiday API website. We make the API call inside an async function, which allows us to use the await
keyword to wait for the response from the server. We have then converted the response to JSON format using the json()
method.
If we open the Console in our browser's developer tools and click the "Get Holidays" button, we should see the holiday data for the United States in January 2022.
Handling the response
The API response contains an array of holiday objects, each with a name, date and type. We can loop through this array and display the data on the page.
async function getHolidays() {
const url = "https://holidayapi.com/v1/holidays?key=YOUR_API_KEY&country=US&year=2022&month=1";
const response = await fetch(url);
const data = await response.json();
const holidays = data.holidays;
const list = document.createElement("ul");
holidays.forEach((holiday) => {
const item = document.createElement("li");
const name = document.createTextNode(holiday.name);
const date = document.createTextNode(holiday.date);
const type = document.createTextNode(holiday.type);
item.appendChild(name);
item.appendChild(document.createElement("br"));
item.appendChild(date);
item.appendChild(document.createElement("br"));
item.appendChild(type);
list.appendChild(item);
});
document.body.appendChild(list);
}
In this example, we have created an unordered list and looped through the holidays
array, creating a list item for each holiday. We have used the createTextNode()
method to add the holiday data to the list item and then appended the item to the list. Finally, we have added the list to the body
of the HTML page.
Conclusion
In this tutorial, we have explored the Holiday API and learned how to make an API call using JavaScript. We have also seen how to handle the response and display the data on the page. You can customize this code to retrieve holiday data for different countries, years and months.