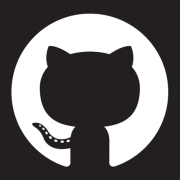
Russian Calendar
CalendarExploring the EGNO Work Calendar API
Have you ever wanted to integrate a calendar into your application, but found the process too complicated? Well, EGNO Work Calendar API is here to help! This public API provides a simple and easy-to-use calendar that can be integrated into your project with just a few lines of code.
Getting started
To get started, you'll need to create an access token for the API. You can do this by following the instructions in the official documentation.
Once you've obtained the access token, you can start exploring the API by making HTTP calls to the endpoints. EGNO Work Calendar API supports both GET
and POST
requests, and returns data in JSON format.
Here are a few examples of how to make requests to the API using JavaScript:
Example 1: Getting the work hours for a specific date
const accessToken = 'YOUR_ACCESS_TOKEN';
const date = '2022-09-01';
fetch(`https://api.egno.works/calendar/hours?date=${date}`, {
method: 'GET',
headers: {
Authorization: `Bearer ${accessToken}`,
},
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the GET
method to retrieve the work hours for a specific date. We're passing the date as a query parameter, and setting the Authorization
header to include our access token. The API will return the work hours data in JSON format, which we're logging to the console.
Example 2: Adding an event to the calendar
const accessToken = 'YOUR_ACCESS_TOKEN';
const event = {
summary: 'Team meeting',
description: 'Weekly team meeting',
start: {
dateTime: '2022-09-01T10:00:00Z',
},
end: {
dateTime: '2022-09-01T11:00:00Z',
},
};
fetch('https://api.egno.works/calendar/events', {
method: 'POST',
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json',
},
body: JSON.stringify(event),
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the POST
method to add an event to the calendar. We're passing the event details as a JSON object in the request body, and setting the Authorization
header to include our access token. The API will add the event to the calendar and return the event data in JSON format, which we're logging to the console.
Wrapping up
EGNO Work Calendar API is a great resource for anyone looking to add a calendar to their application. It's easy to use, requires minimal setup, and provides a reliable and accurate calendar. Give it a try in your next project!