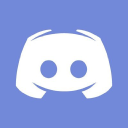
Discord bot API
SocialIntroduction to Discord API
Discord API is a powerful communication platform used by millions of gamers worldwide. It provides a set of APIs to interact with Discord's servers and perform various actions like sending messages, joining servers, creating bots, and much more. In this article, we'll explore the basics of Discord API and discuss how to interact with it using JavaScript.
Getting Started
To start using Discord API, you need to create a Discord account and a Discord developer account. Once you have created these accounts, you can access Discord API documentation from their official website - https://discordapp.com/developers/docs/intro.
Authentication
Discord API requires authentication for all API calls. You can authenticate using your Discord bot token. Make sure to keep your token secure and never share it with anyone.
Here's an example code to authenticate with Discord API using JavaScript:
const Discord = require('discord.js');
const client = new Discord.Client();
client.login('your-token-goes-here');
Sending Messages
One of the most common actions performed using Discord API is sending messages to Discord servers. Here's an example code to send a message to a Discord channel using JavaScript:
const Discord = require('discord.js');
const client = new Discord.Client();
client.login('your-token-goes-here');
client.on('ready', () => {
const channel = client.channels.cache.find(channel => channel.name === 'general');
channel.send('Hello, World!');
});
Retrieving Server Information
You can retrieve server information like server name, member count, etc., using Discord API. Here's an example code to retrieve server information using JavaScript:
const Discord = require('discord.js');
const client = new Discord.Client();
client.login('your-token-goes-here');
client.on('ready', () => {
const guild = client.guilds.cache.find(guild => guild.name === 'My Server');
console.log(`Server Name: ${guild.name}`);
console.log(`Member Count: ${guild.memberCount}`);
});
Conclusion
Discord API is a powerful communication platform that allows you to interact with Discord's servers and perform various actions like sending messages, joining servers, creating bots, and much more. In this article, we discussed the basics of Discord API and provided some example codes in JavaScript to help you get started.
For more information on Discord API and its capabilities, refer to their official documentation.