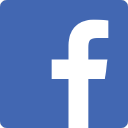
Facebook API
SocialExploring Facebook's Public API with JavaScript
Facebook's Public API is a powerful tool that gives you access to a wide range of data on the platform. With the help of JavaScript, you can interact with this API and build apps that leverage Facebook's data to provide valuable insights and functionality.
Getting Started
To get started, you will need to create a new app on the Facebook Developer Portal and obtain an Access Token. Once you have your Access Token, you can start making requests to the API with JavaScript.
Example 1: Requesting User Information
The following code sends a GET request to the Facebook API to retrieve information about the user:
const accessToken = "{your access token}";
fetch(`https://graph.facebook.com/v13.0/me?access_token=${accessToken}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
This will return a JSON object with information about the user, such as their name, email, and profile picture.
Example 2: Posting a Message
The following code sends a POST request to the Facebook API to post a message on the user's timeline:
const accessToken = "{your access token}";
const message = "Hello, world!";
fetch(`https://graph.facebook.com/v13.0/me/feed?access_token=${accessToken}`, {
method: "POST",
body: JSON.stringify({ message })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
This will post the specified message on the user's timeline.
Example 3: Retrieving Page Posts
The following code sends a GET request to the Facebook API to retrieve the posts on a Facebook Page:
const accessToken = "{your access token}";
const pageId = "{page id}";
fetch(`https://graph.facebook.com/v13.0/${pageId}/feed?access_token=${accessToken}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
This will return a JSON object containing information about the Page's posts, such as their content and creation date.
Conclusion
In this blog post, we explored how to use JavaScript to interact with the Facebook Public API. By leveraging the power of this API, you can build apps that provide valuable insights and functionality to users. With the help of the example codes provided, you can get started quickly and easily. So, go ahead and start experimenting with Facebook's Public API today!