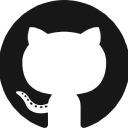
HackerNews
SocialExploring the Hacker News API
Are you a developer looking to build an app that involves fetching data from Hacker News? The Hacker News API is an official, public API that provides access to a large amount of data from Hacker News, including stories, comments, and users.
In this article, we'll take a look at how to get started with the API, and we'll provide a few example code snippets in JavaScript to help you get started.
Getting Started
To get started with the Hacker News API, all you need to do is make HTTP requests to the API endpoints. The base URL for all API requests is https://hacker-news.firebaseio.com/v0/
.
Here are a few example API endpoints:
https://hacker-news.firebaseio.com/v0/topstories.json
: Returns an array of the IDs of the current top stories on Hacker News. Each ID can be used to fetch the full story data from the API.https://hacker-news.firebaseio.com/v0/item/{item-id}.json
: Returns the full data for a specific item, identified by its ID.
Example code snippets
Here are a few example code snippets in JavaScript to help you get started with using the Hacker News API.
Fetching the top stories
fetch('https://hacker-news.firebaseio.com/v0/topstories.json')
.then(response => response.json())
.then(storyIds => {
// Fetch details for each story
storyIds.forEach(storyId => {
fetch(`https://hacker-news.firebaseio.com/v0/item/${storyId}.json`)
.then(response => response.json())
.then(story => {
console.log(story.title);
});
});
});
In this code snippet, we first fetch the array of IDs for the current top stories. Then, for each story ID, we fetch the full story data and log the title to the console.
Fetching comments for a story
const storyId = 12345;
fetch(`https://hacker-news.firebaseio.com/v0/item/${storyId}.json`)
.then(response => response.json())
.then(story => {
// Fetch details for each comment
story.kids.forEach(commentId => {
fetch(`https://hacker-news.firebaseio.com/v0/item/${commentId}.json`)
.then(response => response.json())
.then(comment => {
console.log(comment.text);
});
});
});
In this example code snippet, we fetch the details for a specific story by ID, then fetch the details for each comment on that story and log the text to the console.
Conclusion
The Hacker News API is a powerful tool that provides access to a wealth of data from the popular news aggregation site. By making HTTP requests to the API endpoints, you can retrieve information about stories, comments, and users, and build apps that incorporate that data.
We hope these example code snippets in JavaScript are helpful as you get started with using the Hacker News API. Happy coding!