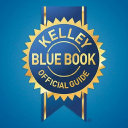
Kelley Blue Book
VehicleGetting started with the Kelley Blue Book API
If you're looking to get information about cars, you might want to check out the Kelley Blue Book API. KBB is a widely-used car valuation and information service in the US. By making use of their public API, you can access this information programmatically.
Getting API keys
To get started with the KBB API, you'll need to sign up on their developer portal and get an API key. Once you have the API key, you'll need to set it as a constant in your JavaScript code.
const apiKey = 'YOUR_API_KEY_HERE';
Accessing the API
Retrieving the current year make model data
fetch(`http://api.kbb.com/v1/vehicle/makeModelYear?year=current&state=USED&utm_campaign=certifiedpreowned&utm_medium=display&utm_source=hanckelroadbannerad&utm_content=survey`, {
headers:{
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error))
This code retrieves the current year make model data for used cars.
Retrieving value range for a given year, make and model
fetch(`http://api.kbb.com/v1/vehicle/value/${year}/${make}/${model}/condition/${condition}?mileage=${mileage}&zipcode=${zip}`, {
headers:{
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error))
This code retrieves the value range for a given year, make and model of a car, for a specific mileage and zip code.
Retrieving specific vehicle data
fetch(`http://api.kbb.com/v1/vehicle/${vin}`, {
headers:{
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error))
This code retrieves a specific vehicle data by its VIN number.
Conclusion
The Kelley Blue Book API is an extremely useful tool for developers looking to access information about cars in the US. By following the examples in this post, you can easily get started with the KBB API and start building your own applications.