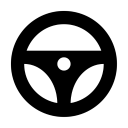
Smartcar
VehicleIntroduction to Smartcar API
Smartcar provides an API to simplify the integration of connected cars into third-party applications. The API allows to query information about the vehicle, such as fuel level, odometer, and location, as well as control certain functions, such as locking and unlocking doors, and starting and stopping the engine.
To use the Smartcar API, you need to create an application and authenticate the user with their vehicle. This can be done through the OAuth 2.0 protocol.
How to use the Smartcar API
The Smartcar API exposes various endpoints to interact with the vehicle. Here are some examples of how to use the API with JavaScript:
Authentication
To authenticate the user, you need to redirect them to Smartcar's authorization page:
const authUrl = smartcar.getAuthUrl({
scope: ['read_vehicle_info', 'control_security'],
redirectUri: 'https://example.com/callback'
});
window.location.href = authUrl;
After the user grants permission, Smartcar will redirect them back to the redirectUri
with a one-time authorization code. You can use the code to exchange it for an access token:
smartcar.exchangeCode(code)
.then(function(access) {
console.log(access.accessToken);
});
You can then use the accessToken
to make requests to the Smartcar API.
Vehicle information
To retrieve information about the vehicle, you can call the vehicle.info()
method:
vehicle.info().then(function(data) {
console.log(data);
});
The data
object will contain information about the vehicle, such as make, model, and VIN.
Lock and unlock
To lock or unlock the vehicle, you need to call the vehicle.lock()
or vehicle.unlock()
method:
vehicle.lock().then(function(response) {
console.log(response);
});
The response
object will contain information about whether the command was successful.
Start and stop
To start or stop the engine, you need to call the vehicle.startEngine()
or vehicle.stopEngine()
method:
vehicle.startEngine().then(function(response) {
console.log(response);
});
The response
object will contain information about whether the command was successful.
Conclusion
The Smartcar API provides a simple and convenient way to interact with connected cars. With just a few lines of JavaScript code, you can retrieve information about the vehicle and control certain functions. To learn more about the API, check out the official documentation.