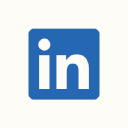
LinkedIn REST-API
SocialThe foundation of all digital integrations with LinkedIn. The REST API is the heart of all programatic interactions with LinkedIn. All other methods of interacting, such as the JavaScript and Mobile SDKs, are simply wrappers around the REST API to provide an added level of convienence for developers. As a result, even if you are doing mobile or JavaScript development, it's still worth taking the time to familiarize yourself with how the REST API works and what it can do for you..
📚 Documentation & Examples
Everything you need to integrate with LinkedIn REST-API
🚀 Quick Start Examples
// LinkedIn REST-API API Example
const response = await fetch('https://developer.linkedin.com/docs/rest-api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Accessing LinkedIn using LinkedIn REST API
LinkedIn provides access to its data with the help of their REST API. LinkedIn REST API is an interface that helps developers to get access to the LinkedIn data from within their own applications.
The LinkedIn REST API allows you to interact with your network data, companies, groups, and more. It also provides you with a way to access your own LinkedIn profile data.
To access LinkedIn REST API first, you need to create an app on the LinkedIn developer portal. You can then use the API key and API secret provided by LinkedIn to authenticate your requests to the LinkedIn server.
Setting Up the LinkedIn App
- Go to the LinkedIn Developer Portal
- Sign in with your LinkedIn credentials
- Select "Create App" from the dropdown menu on the top right corner
- Fill out the necessary information about the app and agree to the terms and conditions to proceed
- Once you have created the app, you will be provided with an API key and API secret. Keep them secure, as you will need them to authenticate your requests to the LinkedIn server.
Accessing LinkedIn REST API using JavaScript
Now that you have the API key and API secret, you can use the JavaScript language to access LinkedIn REST API. Here are some examples of how you can use the LinkedIn REST API using JavaScript:
Example 1: Retrieving Your Own Profile Information
const apiKey = '[API KEY]';
const secretKey = '[SECRET KEY]';
const url = `https://api.linkedin.com/v2/me?projection=(id,firstName,lastName,profilePicture(displayImage~:playableStreams))`;
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.setRequestHeader('Authorization', `Bearer ${access_token}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response);
}
}
xhr.send();
Example 2: Retrieving a Company's Information
const apiKey = '[API KEY]';
const secretKey = '[SECRET KEY]';
const companyId = '[COMPANY ID]';
const url = `https://api.linkedin.com/v2/companies/${companyId}?projection=(id,name,logoV2(original~:url))`;
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.setRequestHeader('Authorization', `Bearer ${access_token}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response);
}
}
xhr.send();
Example 3: Searching for People on LinkedIn
const apiKey = '[API KEY]';
const secretKey = '[SECRET KEY]';
const keywords = 'developer';
const count = 10;
const url = `https://api.linkedin.com/v2/search?q=title:${keywords}&facetCurrentCompany=3263&facetGeoRegion=us%3A0&facetNetwork=%5BF%5D&count=${count}&start=0&qp=context&projection=(id,firstName,lastName,vanityName,profilePicture(displayImage~:playableStreams),headline,positions)`;
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.setRequestHeader('Authorization', `Bearer ${access_token}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response);
}
}
xhr.send();
LinkedIn REST API provides a lot of functionality to get rich, professional user data, company information, messaging API, and more. These were just a few examples to get you started. Please visit the LinkedIn Developer Portal for more information about the LinkedIn REST API.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes