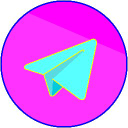
Madeline Proto API
SocialGetting started with MadelineProto API in JavaScript
If you're looking to use MadelineProto API with your JavaScript application, then this handy guide will help you get started. MadelineProto API, developed by Telegram, provides seamless integration with Telegram messenger app. This API can be used for various messaging-related functionalities, including sending and receiving messages, media sharing, chat customizations, and more.
Prerequisites
Before getting started, you should have some basic knowledge of:
- JavaScript programming language
- RESTful API and concepts
- HTTP requests and responses
Additionally, you might want to install a package manager like npm
to manage your project dependencies more effectively.
Getting API key
To use MadelineProto API, you will need an API key (also known as an access token). To get a key:
- Visit https://my.telegram.org/apps and sign in to your Telegram account.
- Click on the "Create Application" button and fill out the necessary fields.
- Once the application is created, you will receive an API key that you should keep confidential.
Installation
To install MadelineProto, you can use npm:
$ npm install madelineproto
You can also use yarn:
$ yarn add madelineproto
Examples
Sending a message
const MadelineProto = require('madelineproto');
const client = new MadelineProto({
"app": {
"api_id": "API_ID",
"api_hash": "API_HASH"
}
});
(async () => {
await client.start();
await client.sendMessage({peer: 'username', message: 'Hello World!'});
})();
Getting the list of chats
const MadelineProto = require('madelineproto');
const client = new MadelineProto({
"app": {
"api_id": "API_ID",
"api_hash": "API_HASH"
}
});
(async () => {
await client.start();
const chats = await client.getChats({limit: 10});
console.log(chats);
})();
Uploading a file
const MadelineProto = require('madelineproto');
const client = new MadelineProto({
"app": {
"api_id": "API_ID",
"api_hash": "API_HASH"
}
});
(async () => {
await client.start();
const file = await client.uploadFile('example.png');
console.log(file);
})();
Creating a new channel
const MadelineProto = require('madelineproto');
const client = new MadelineProto({
"app": {
"api_id": "API_ID",
"api_hash": "API_HASH"
}
});
(async () => {
await client.start();
const channel = await client.invokeApi('channels.createChannel', {
'broadcast': false,
'megagroup': false,
'title': 'test_channel',
'about': 'This is a test channel created via MadelineProto API'
});
console.log('Channel ID:', channel.chats[0].id);
})();
Conclusion
MadelineProto API offers a ton of functionalities that can be integrated into your JavaScript application with ease. With proper implementation, you can provide users with a seamless messaging experience, making use of the powerful functionality offered by this API.