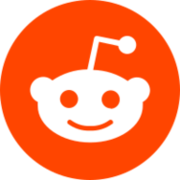
Homepage of the internet. Many endpoints on reddit use the same protocol for controlling pagination and filtering. These endpoints are called Listings and share five common parameters: after / before, limit, count, and show. Listings do not use page numbers because their content changes so frequently. Instead, they allow you to view slices of the underlying data. Listing JSON responses contain after and before fields which are equivalent to the "next" and "prev" buttons on the site and in combination with count can be used to page through the listing.
📚 Documentation & Examples
Everything you need to integrate with Reddit
🚀 Quick Start Examples
// Reddit API Example
const response = await fetch('https://www.reddit.com/dev/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring Reddit's Public API
Reddit's public API is a powerful tool for interacting with the platform's vast database of posts and comments. The API provides a broad range of endpoints that allow developers to create their own applications that can search, sort, and analyze Reddit data with ease.
Getting Started
The first step to using the Reddit API is to register for an account on the Reddit website. This will allow you to get an API key which is required for all API requests.
Once you have a Reddit account, you can find your API key by navigating to the Apps & API section of your account settings. This will show you all of the authorized apps and API keys associated with your account.
Endpoint Examples
Here are some examples of how to use various endpoints of the Reddit API in JavaScript:
Getting Hot Posts
fetch("https://www.reddit.com/r/javascript/hot.json")
.then(response => response.json())
.then(data => {
// Use data to display hot posts
console.log(data);
})
.catch(error => {
console.error(error);
});
Searching for Posts
fetch("https://www.reddit.com/search.json?q=javascript")
.then(response => response.json())
.then(data => {
// Use data to display search results
console.log(data);
})
.catch(error => {
console.error(error);
});
Getting Comments for a Post
fetch("https://www.reddit.com/r/javascript/comments/oohk3g.json")
.then(response => response.json())
.then(data => {
// Use data to display comments
console.log(data);
})
.catch(error => {
console.error(error);
});
Getting User Info
fetch("https://www.reddit.com/api/v1/me.json", {
headers: {
"Authorization": "bearer YOUR_API_KEY_HERE"
}
})
.then(response => response.json())
.then(data => {
// Use data to display user info
console.log(data);
})
.catch(error => {
console.error(error);
});
Conclusion
The Reddit API is a valuable tool for developers who want to build applications that interact with the popular social media platform. By using the examples above, you can get started with exploring the API and building your own projects. Be sure to check out the full documentation on the Reddit website for a complete list of endpoints and their usage.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes