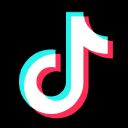
TikTok
SocialThe TikTok User Info and Video Posts API is an essential tool for developers looking to integrate user information and video content from the popular TikTok platform into their applications. By leveraging this API, you can seamlessly retrieve a user's profile details, such as their username, profile picture, and bio, alongside accessing their video posts. This opens up a myriad of possibilities for user engagement and content interaction, enhancing the overall user experience while promoting user-generated content directly within your application. With a robust authentication mechanism in place, the API ensures that user data is accessed securely and efficiently, fostering trust and reliability in your integration.
Utilizing this API comes with several benefits:
- Access complete user profiles and their TikTok videos effortlessly.
- Enhance user engagement by displaying personalized content within your app.
- Foster a sense of community by integrating user-generated videos.
- Save development time with well-documented endpoints and examples.
- Maintain high security with OAuth2 authentication protocol for data access.
Here’s a simple JavaScript example demonstrating how to call the TikTok User Info and Video Posts API:
const fetchUserInfo = async (userId, accessToken) => {
const url = `https://api.tiktok.com/user/${userId}/info`;
const response = await fetch(url, {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
if (response.ok) {
const data = await response.json();
console.log('User Info:', data);
} else {
console.error('Error fetching user info:', response.statusText);
}
};
// Example usage
const userId = '1234567890'; // Replace with actual user ID
const accessToken = 'your_access_token_here'; // Replace with your access token
fetchUserInfo(userId, accessToken);