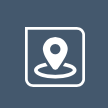
TravelBriefing API
SocialSimple Guide to Using the TravelBriefing API
Are you looking for an API that can provide you with comprehensive information about different countries? Check out the TravelBriefing API, a free and user-friendly API service that offers valuable insights into a country's visa and passport requirements, tourism and health information, and more.
The TravelBriefing API is available in JSON format, and thanks to its well-organized documentation and clear examples, it's easy to integrate it into your applications. In this guide, we'll go over some of the most important features of the TravelBriefing API and share some examples of how to use it with JavaScript.
Getting Started
First, you'll need to sign up for a free API key from the TravelBriefing website: https://travelbriefing.org/api. Once you have your API key, you can start using the API by sending HTTP requests to the TravelBriefing server.
Here's an example of how you can send a request to the TravelBriefing API using JavaScript's Fetch API:
fetch('https://travelbriefing.org/api/your_api_key/country?cc=NL')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the example above, we're requesting information about the Netherlands by sending a GET request to the TravelBriefing API. We then use the .json()
method to parse the response into JSON format and log it to the console.
API Endpoint
The TravelBriefing API is designed around a single endpoint, the /country
endpoint. This endpoint accepts several parameters, including the country code (cc
) and the API key (api_key
).
Here's an example of how you can use the /country
endpoint to retrieve information about a specific country:
fetch(`https://travelbriefing.org/api/your_api_key/country?cc=ES`)
.then(response => response.json())
.then(data => {
// Do something with the response data
})
.catch(error => console.error(error));
In the example above, we're requesting information about Spain by passing the cc
parameter with value "ES" to the API's country
endpoint. Replace "your_api_key"
with your own API key.
Query Parameters
The TravelBriefing API's /country
endpoint accepts several query parameters that you can use to filter and refine the API's response:
cc
(required)
The country code of the country you want to get information about.
format
The format of the API's response. You can choose between the json
and yaml
formats.
version
The API version. The default version is 3.
Example
fetch(`https://travelbriefing.org/api/your_api_key/country?cc=US&format=yaml&version=2`)
.then(response => response.json())
.then(data => {
// Do something with the response data
})
.catch(error => console.error(error));
In this example, we're specifying that we want the API to return the response in YAML format and that we want to use version 2 of the API.
Conclusion
In this guide, we've explored the basics of the TravelBriefing API and how to use it with JavaScript. With its easy-to-use interface and detailed documentation, the TravelBriefing API is an excellent choice for anyone looking to gain insights into other countries' travel requirements and general information. Try using TravelBriefing's API the next time you need to add country-specific information to your app, and let us know what you think!