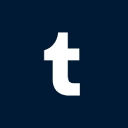
Tumblr API
SocialA Beginner's Guide to Using the Tumblr API
Looking to create a Tumblr app or integrate Tumblr into your website? The Tumblr API is a great way to access Tumblr's massive library of content and user data. In this guide, we'll walk you through the basics of using the Tumblr API and provide sample code in JavaScript for various API endpoints.
Step 1: Get an API Key
Before you start working with the Tumblr API, you'll need to obtain an API key. Head over to the Tumblr API website and click on "Get API Key". You'll then need to fill out a form with some basic information about your app and agree to the terms of service.
Once you've completed the form, you'll be given a consumer key and a consumer secret. These will be used to authenticate your app when making API requests.
Step 2: Make Your First API Request
Now that you've got your API key, it's time to make your first API request! The Tumblr API is a RESTful API that uses HTTP requests to communicate with the server. To make a request, you'll need to construct a URL that includes the endpoint you want to access and any necessary parameters.
Let's start by retrieving a list of the most recent posts on a Tumblr blog. Here's the code you'll need in JavaScript:
const consumerKey = 'YOUR_CONSUMER_KEY';
const consumerSecret = 'YOUR_CONSUMER_SECRET';
const blogName = 'YOUR_BLOG_NAME';
const apiUrl = `https://api.tumblr.com/v2/blog/${blogName}.tumblr.com/posts`;
fetch(`${apiUrl}?api_key=${consumerKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're using the fetch()
method to make a GET request to the /posts
endpoint of the specified blog. We've also included our consumer key as a query parameter in the URL.
When the server responds to our request, we'll receive a JSON object that contains information about the blog's 20 most recent posts. You can use this data to display the posts on your own website or manipulate it in other ways.
Step 3: Explore the API Documentation
The Tumblr API provides a wide range of endpoints that you can use to access different types of content and data. To learn more about what's available, head over to the Tumblr API documentation.
From there, you can browse the various endpoints and check out example responses to see what kind of data you can expect to receive. You'll also find information on any required parameters and authentication methods.
Conclusion
The Tumblr API is a powerful tool for accessing Tumblr content and data. By following these steps and exploring the API documentation, you should be well on your way to building your own Tumblr app or integrating Tumblr into your website.