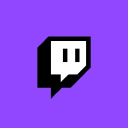
Twitch
SocialGame Streaming API. Interactive experiences that work as a stream overlay or as a panel. As simple as writing a web app. Create interactive experiences within Twitch chat, which can be used to take requests, moderate content and more. Use Twitch data and Identity tools to enhance your game or connect with your app.
π Documentation & Examples
Everything you need to integrate with Twitch
π Quick Start Examples
// Twitch API Example
const response = await fetch('https://dev.twitch.tv/docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Are you interested in creating a Twitch app? Twitch, a popular video game streaming platform, provides a comprehensive API documentation that enables developers to access data and functionalities on their platform. In this blog post, we will explore Twitch API, along with some example code snippets in JavaScript, which can be utilized to create your own Twitch-powered app.
Twitch API
Twitch API provides authenticated access to Twitch data, functionalities, and streaming capabilities. Developers can use Twitch API to retrieve information about streams, channels, users, games, and more. Twitch API supports both REST and WebSockets protocols. The documentation also includes guidelines for authenticating your app, rate limiting, and troubleshooting common issues.
Authentication
Before we can start using the Twitch API, we need to authenticate our app to ensure that the userβs data is secure. To authenticate an app, we need to obtain an OAuth token. Tokens can be generated either through an authorization code or a client credential flow.
Authorization code flow
The authorization code flow is the preferred method to generate an OAuth token. It involves two primary steps - obtaining an authorization code and using the code to exchange it for an access token. Here is an example code snippet to generate an OAuth token using authorization code flow -
const clientId = "YOUR_CLIENT_ID";
const redirectUri = "YOUR_REDIRECT_URI";
const scope = "user_read";
const responseType = "code";
// send the user to Twitch's authorization page
const authUrl = `https://id.twitch.tv/oauth2/authorize?client_id=${clientId}&redirect_uri=${redirectUri}&response_type=${responseType}&scope=${scope}`;
// upon successful authorization, Twitch will redirect the user to `redirectUri` with an authorization code
const authorizationCode = "";
// exchange the authorization code for an access token
const getAccessToken = async () => {
const clientSecret = "YOUR_CLIENT_SECRET";
const grantType = "authorization_code";
const response = await fetch("https://id.twitch.tv/oauth2/token", {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded",
},
body: new URLSearchParams({
client_id: clientId,
client_secret: clientSecret,
code: authorizationCode,
grant_type: grantType,
redirect_uri: redirectUri,
}),
});
const data = await response.json();
const accessToken = data.access_token;
console.log(accessToken);
};
Client credential flow
The client credential flow is suitable for server-side apps that do not require userβs permission to access Twitch data. It involves generating a client ID and client secret for your Twitch app and using them to obtain an access token. Here is an example code snippet to generate an OAuth token using client credential flow -
const clientId = "YOUR_CLIENT_ID";
const clientSecret = "YOUR_CLIENT_SECRET";
const grantType = "client_credentials";
// obtain an access token using client id and client secret
const getAccessToken = async () => {
const response = await fetch("https://id.twitch.tv/oauth2/token", {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded",
},
body: new URLSearchParams({
client_id: clientId,
client_secret: clientSecret,
grant_type: grantType,
}),
});
const data = await response.json();
const accessToken = data.access_token;
console.log(accessToken);
};
REST API example
Once we have an OAuth token, we can use it to make API requests. Here is an example code snippet to retrieve the details of a particular Twitch channel -
const accessToken = "YOUR_ACCESS_TOKEN";
const channelId = "CHANNEL_ID";
// retrieve channel details
const getChannelDetails = async () => {
const response = await fetch(`https://api.twitch.tv/helix/channels?broadcaster_id=${channelId}`, {
headers: {
"Client-ID": clientId,
"Authorization": `Bearer ${accessToken}`
},
});
const data = await response.json();
console.log(data);
};
WebSockets API example
Twitch API also supports real-time notification using WebSockets protocol. Here is an example code snippet to connect to Twitch IRC chat using WebSockets -
const socket = new WebSocket("wss://irc-ws.chat.twitch.tv:443");
const authToken = "YOUR_AUTH_TOKEN";
const username = "YOUR_USERNAME";
const channel = "CHANNEL_NAME";
socket.addEventListener("open", () => {
// authenticate the user with Twitch IRC server
socket.send(`PASS ${authToken}`);
socket.send(`NICK ${username}`);
// join the channel
socket.send(`JOIN #${channel}`);
});
socket.addEventListener("message", (event) => {
console.log(event.data);
});
Conclusion
Twitch API provides a powerful toolset for developers to build Twitch-powered apps. In this blog post, we explored Twitch API, along with some example code snippets in JavaScript. These examples include authentication, REST API usage, and WebSockets integration. By leveraging Twitch API, developers can create engaging experiences for the Twitch community.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes