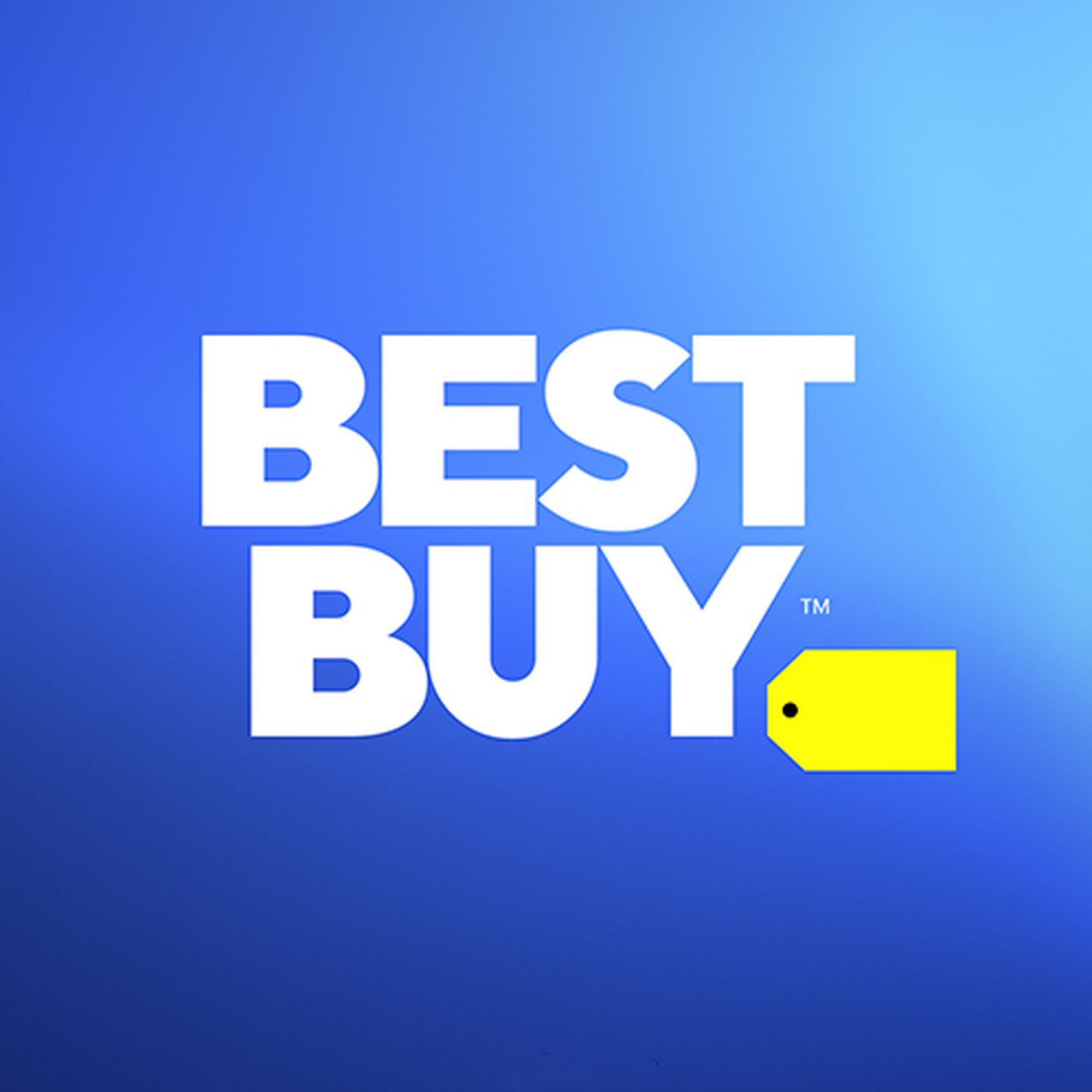
Best Buy
ShoppingBest Buy API: A Quick Guide with JavaScript Examples
The Best Buy API allows developers to access Best Buy's product information, store information, purchasing options, and more. This API is publicly available and provides clear and concise documentation. In this post, we will guide you through the API's overview and offer several examples featuring JavaScript.
Overview
The Best Buy API provides RESTful web services with JSON-formatted responses. In order to access the API, you will need an API key that can be obtained from the Best Buy Developer Portal. Currently, there is only one version of the Best Buy API, v1.
JavaScript Examples
1. Search for a Product
fetch(`https://api.bestbuy.com/v1/products(search=samsung)?apiKey=${api_key}&format=json`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.log(error));
This code makes a search query for "samsung" products and retrieves the associated data in JSON format. Replace api_key
with your own API key.
2. Retrieve Product Details
fetch(`https://api.bestbuy.com/v1/products(productId=6364253)?apiKey=${api_key}&format=json`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.log(error));
This code retrieves the product with ID "6364253" and its details. Replace api_key
with your own API key.
3. Retrieve Store Locations
fetch(`https://api.bestbuy.com/v1/stores(area(55416,25))?apiKey=${api_key}&format=json`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.log(error));
This code retrieves stores within 25 miles of the ZIP code "55416". Replace api_key
with your own API key.
4. Retrieve Top Deals
fetch(`https://api.bestbuy.com/v1/products(onSale=true)?apiKey=${api_key}&format=json&pageSize=10&sort=bestSellingRank.asc`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.log(error));
This code retrieves the top 10 products on sale and sorts them by their best selling rank in ascending order. Replace api_key
with your own API key.
These examples are just a glimpse of what the Best Buy API can do. With the help of JavaScript, you can access a wide range of data and functionalities. We hope that this guide has been helpful in getting you started with the Best Buy API!