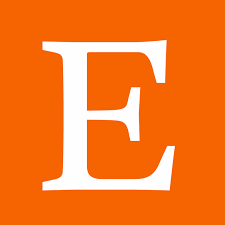
Etsy
ShoppingEtsy is a popular e-commerce platform that allows individuals and businesses to buy and sell handmade, vintage, and unique items. The Etsy Developers API provides a set of tools that allows developers to manage shops and interact with listings on the platform. With these tools, developers can access a wide range of features, including the ability to create and edit listings, manage shop settings, and retrieve order information. Whether you're a developer building an e-commerce app or a shop owner looking to streamline your workflow, the Etsy Developers API is a valuable resource that can help you achieve your goals. So why not check it out today and start exploring the possibilities?
📚 Documentation & Examples
Everything you need to integrate with Etsy
🚀 Quick Start Examples
// Etsy API Example
const response = await fetch('https://www.etsy.com/developers/documentation/getting_started/api_basics', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring Etsy's Public API
Etsy is a popular online marketplace where you can shop for unique and handmade items from independent sellers. However, what you may not know is that Etsy also offers a public API that can be used to build applications, websites, or tools that leverage Etsy's platform. In this blog post, we'll explore some of the basics of using Etsy's public API and provide some example code in JavaScript.
Getting Started
Before using Etsy's API, you'll first need to create an Etsy developer account, and then register an application to obtain your API key. You can find detailed instructions on how to do this in the Etsy Developer Documentation.
Once you have your API key, you can start making API requests to Etsy's servers. There are many endpoints that you can use to retrieve data from Etsy, such as /listings
, /shops
, /users
, /carts
, and more. You can see a full list of available endpoints in the Etsy API Reference.
Example Code
Below is some example code in JavaScript that demonstrates how you can use the fetch
API to make an API request to Etsy's /listings
endpoint and retrieve information about the first 10 listings. Note that you'll need to replace YOUR_API_KEY
with your actual API key.
const API_KEY = 'YOUR_API_KEY';
const API_URL = `https://openapi.etsy.com/v2/listings/active?api_key=${ API_KEY }&limit=10`;
fetch(API_URL)
.then(response => response.json())
.then(data => {
// Process the data you received from Etsy here
console.log(data);
})
.catch(error => console.error(error));
In this example code, we're using the fetch
API to make a GET request to the /listings/active
endpoint, and we're passing our API key and a query parameter for limiting the number of listings to return (in this case, 10) in the URL. When the request is successful, the response data is returned as a JSON object, which we can then process as needed.
Here's another example that uses the /shops/:shop_id
endpoint to retrieve information about a specific Etsy shop by its ID:
const API_KEY = 'YOUR_API_KEY';
const SHOP_ID = '12345678'; // Replace with a valid shop ID
const API_URL = `https://openapi.etsy.com/v2/shops/${ SHOP_ID }?api_key=${ API_KEY }`;
fetch(API_URL)
.then(response => response.json())
.then(data => {
// Process the data you received from Etsy here
console.log(data);
})
.catch(error => console.error(error));
In this example, we're using a template literal to interpolate the shop ID into the URL for the /shops/:shop_id
endpoint, and passing our API key in the URL as before.
Conclusion
In this blog post, we've covered some basic information about the Etsy public API and provided some example code in JavaScript for making API requests to various endpoints. By using Etsy's API, you can build applications and tools that leverage Etsy's platform and provide unique and valuable experiences for your users.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes