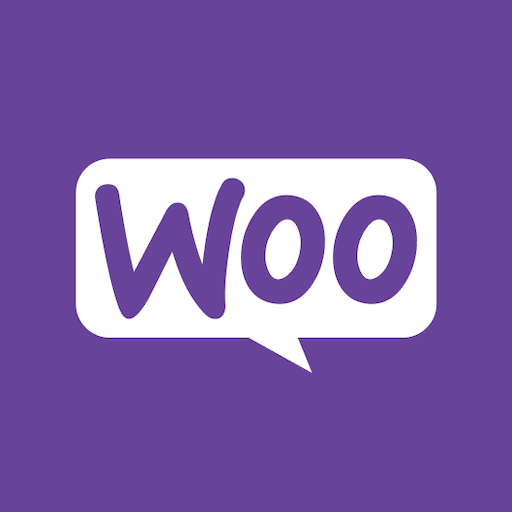
WooCommerce
ShoppingExploring the WooCommerce REST API with JavaScript
WooCommerce is a popular e-commerce platform that allows website owners to create online stores with ease. One of the best features of WooCommerce is its powerful REST API, which let developers connect to WooCommerce-powered websites and build custom solutions.
In this blog post, we will explore the WooCommerce REST API and show you some examples of how to use it using JavaScript.
Getting started with the WooCommerce REST API
Before we do anything else, we need to have an understanding of how to authenticate and connect with the WooCommerce API. You need to create API keys first. Once you have that, you can use it to authenticate your JavaScript requests.
Here's an example code snippet that shows how to authenticate with the WooCommerce API using JavaScript:
const consumerKey = 'your_consumer_key';
const consumerSecret = 'your_consumer_secret';
const apiUrl = 'https://yourstore.com/wp-json/wc/v3';
const WooCommerce = new WooCommerceAPI({
url: apiUrl,
consumerKey: consumerKey,
consumerSecret: consumerSecret,
version: 'wc/v3',
queryStringAuth: true
});
You need to replace your_consumer_key
, your_consumer_secret
, and https://yourstore.com/wp-json/wc/v3
with your own consumer key, consumer secret, and website URL, respectively.
Once you have authenticated, you can now start using the WooCommerce REST API in your JavaScript code.
Retrieving Products
One of the most common operations when working with an e-commerce site is retrieving products from the store. The WooCommerce REST API makes it easy to do this.
Here's an example code snippet that shows how to retrieve a list of products using the WooCommerce REST API:
WooCommerce.get('products', function(err, data, res) {
console.log(data);
});
This code will retrieve all products from your WooCommerce-powered website and log them to the console.
Creating a product
Creating a new product on your WooCommerce store using the REST API is also very simple.
const data = {
name: 'New Product',
type: 'simple',
regular_price: '15.99',
description: 'A new product that has just been added via the API'
};
WooCommerce.post('products', data, function(err, data, res) {
console.log(data);
});
This code will create a new product called 'New Product' with a regular price of $15.99, and a description of 'A new product that has just been added via the API' to your WooCommerce-powered store.
Updating a product
Updating a product using the WooCommerce REST API is also straightforward.
const data = {
name: 'Updated Product',
regular_price: '19.99',
description: 'This product was updated via the API'
};
WooCommerce.put('products/123', data, function(err, data, res) {
console.log(data);
});
This code will update an existing product with the ID of 123, changing its name to 'Updated Product', its regular price to $19.99, and its description to 'This product was updated via the API'.
Conclusion
In conclusion, we've seen how easy it is to work with the WooCommerce REST API and JavaScript. You can use this API to retrieve products, create new products, and update existing products on your WooCommerce-powered website.
Happy coding!