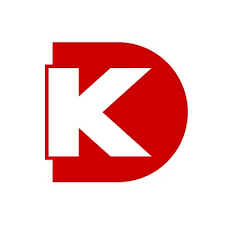
Digi-Key
ShoppingDigi-Key API Solutions: A Comprehensive Guide
Digi-Key is a leading provider of electronic components, providing over 8 million components from more than 1,300 manufacturers. Digi-Key's API solutions make it possible to integrate their vast inventory directly into your software. Whether you're building an e-commerce platform, manufacturing automation software, or an electronics project, Digi-Key APIs could be the answer to your needs.
In this blog post, we'll guide you through Digi-Key's API solutions, including how to access them and examples of how to use the Digi-Key RESTful APIs with JavaScript.
Accessing the Digi-Key API
To access the Digi-Key APIs, you'll need to obtain an API key. You can sign up for a free account on the Digi-Key website, and once you're logged in, navigate to the API Solutions page. From there, you'll be able to view all of Digi-Key's API offerings, as well as generate a new API key.
Using the API with JavaScript
The Digi-Key API can be used with any programming language that can make RESTful API calls. In this guide, we'll show you how to use JavaScript to interact with the Digi-Key API via HTTP GET requests.
Example Code: Search for a Part Number
Suppose you want to look up a specific component by part number. The following code demonstrates how to send a HTTP GET request to the Digi-Key API to fetch the product information for a specific part number:
const partNumber = 'MCP9808T-BE/MC'; // replace this with the desired part number
fetch(`https://api.digikey.com/v1/productsearch?keywords=${partNumber}`, {
headers: {
'x-ibm-client-id': 'YOUR_API_KEY',
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Code: Search for Products by Keyword
If you're looking for parts that match a specific keyword, you can query the Digi-Key API by using a keyword search. The following code demonstrates how to search for products using a keyword:
const keyword = 'resistor'; // replace this with the desired search term
fetch(`https://api.digikey.com/v1/products?keywords=${keyword}`, {
headers: {
'x-ibm-client-id': 'YOUR_API_KEY',
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Code: Retrieve Product Details by Part Number
If you have a part number and want to retrieve all the details about a product, you can query the Digi-Key API by using the part number search. The following code demonstrates how to get all the details about a product:
const partNumber = 'MCP9808T-BE/MC'; // replace this with the desired part number
fetch(`https://api.digikey.com/v1/products/${partNumber}`, {
headers: {
'x-ibm-client-id': 'YOUR_API_KEY',
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Conclusion
Digi-Key's API solutions provide a powerful way to access millions of electronic components and integrate them into your software projects. In this guide, we've shown you how to get started with the Digi-Key API and provided some examples of how to use it with JavaScript. We hope this has been a useful resource and encourage you to explore Digi-Key's API solutions for your own projects.