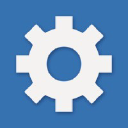
Octopart
ShoppingThe Electronic Part Data API is a crucial tool for professionals in manufacturing, design, and sourcing, enabling access to a comprehensive database of electronic components. This API offers detailed information about parts, including specifications, pricing, availability, and supplier options, empowering engineers and buyers to make informed decisions quickly. By integrating this API, businesses can streamline their sourcing processes, enhance product design efficiency, and reduce time spent on research, ultimately driving innovation and cost savings in their projects. Additionally, the API is designed to be user-friendly, accommodating both experienced developers and those new to API integration, making it an essential resource for any organization working with electronic components.
Utilizing the Electronic Part Data API comes with numerous benefits, including:
- Access to extensive databases of electronic parts and components.
- Real-time information on pricing and availability from multiple suppliers.
- Enhanced efficiency in the design and sourcing processes.
- Streamlined workflows for manufacturers and designers through easy integration.
- Up-to-date technical specifications aiding in better product development.
Here’s a JavaScript code example for calling the API:
const fetch = require('node-fetch');
async function getElectronicPartData(partNumber) {
const apiKey = 'YOUR_API_KEY'; // Replace with your actual API key
const url = `https://octopart.com/api/v4/parts/search?q=${partNumber}&apikey=${apiKey}`;
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Fetch error:', error);
}
}
getElectronicPartData('LM358');