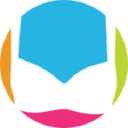
Bratabase
ShoppingGetting Started with the Bratabase Public API
The Bratabase Public API allows you to retrieve data from their vast collection of bra products. In order to use the API, you will need to create an account on their developer platform. Once you've created an account, you will be provided with an API key that can be used to authenticate your requests.
How to Use the Bratabase Public API
Before starting, make sure that you have Node.js installed.
Installation
The first thing you'll need to do is install the 'axios' module. You can do this by running the following command in the terminal:
$ npm install axios
Example Code
Below are some examples of how to use the Bratabase Public API in JavaScript:
Get a List of Bra Brands
const axios = require('axios');
axios.get('https://api.bratabase.com/brands/', {
headers: {
'Authorization': 'Token {{YOUR_API_KEY}}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Get a Specific Bra Brand
const axios = require('axios');
axios.get('https://api.bratabase.com/brands/{{BRAND_ID}}/',{
headers: {
'Authorization': 'Token {{YOUR_API_KEY}}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Get a List of Bra Models
const axios = require('axios');
axios.get('https://api.bratabase.com/models/', {
headers: {
'Authorization': 'Token {{YOUR_API_KEY}}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Get a Specific Bra Model
const axios = require('axios');
axios.get('https://api.bratabase.com/models/{{MODEL_ID}}/',{
headers: {
'Authorization': 'Token {{YOUR_API_KEY}}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Conclusion
In conclusion, the Bratabase Public API is a powerful tool for retrieving data about bra products. By following this short guide, you should now be able to get started using the API in your own projects. If you have any questions or need further assistance, please refer to the official documentation on the Bratabase developer platform.