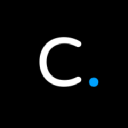
Cloudmersive Natural Language Processing
Text AnalysisA Guide to the Cloudmersive NLP API
The Cloudmersive NLP API is a powerful tool to analyze and process natural language. With this API, you can extract keywords and entities from a text, determine sentiment and emotion, and perform text matching. In this article, we will walk through some examples of using the Cloudmersive NLP API using JavaScript.
Installation
To use the Cloudmersive NLP API, you will need to install the cloudmersive-nlp-api-client
package using npm:
npm install cloudmersive-nlp-api-client
Example 1: Extracting Keywords
The following code snippet demonstrates how to extract keywords from a given text using the Cloudmersive NLP API:
const CloudmersiveNLPApi = require('cloudmersive-nlp-api-client');
const defaultClient = CloudmersiveNLPApi.ApiClient.instance;
const APIKey = 'YOUR_API_KEY';
// Set API key
defaultClient.authentications['Apikey'].apiKey = APIKey;
const apiInstance = new CloudmersiveNLPApi.KeywordExtractorApi();
const inputText = 'This is a sample text to extract keywords from.';
apiInstance.keywordExtractorExtract(inputText, (error, data) => {
if (error) {
console.error(error);
} else {
console.log('Keywords: ' + data.keywords);
}
});
Replace YOUR_API_KEY
with your actual API key.
Example 2: Determining Sentiment
The following code snippet demonstrates how to determine the sentiment of a given text using the Cloudmersive NLP API:
const CloudmersiveNLPApi = require('cloudmersive-nlp-api-client');
const defaultClient = CloudmersiveNLPApi.ApiClient.instance;
const APIKey = 'YOUR_API_KEY';
// Set API key
defaultClient.authentications['Apikey'].apiKey = APIKey;
const apiInstance = new CloudmersiveNLPApi.SentimentAnalysisApi();
const inputText = 'This is a positive text.';
apiInstance.sentimentAnalysisSentimentPost(inputText, (error, data) => {
if (error) {
console.error(error);
} else {
console.log('Sentiment: ' + data.sentiment);
}
});
Replace YOUR_API_KEY
with your actual API key.
Example 3: Text Matching
The following code snippet demonstrates how to perform text matching using the Cloudmersive NLP API:
const CloudmersiveNLPApi = require('cloudmersive-nlp-api-client');
const defaultClient = CloudmersiveNLPApi.ApiClient.instance;
const APIKey = 'YOUR_API_KEY';
// Set API key
defaultClient.authentications['Apikey'].apiKey = APIKey;
const apiInstance = new CloudmersiveNLPApi.StringOperationsApi();
const inputText = 'This is a text to match.';
const pattern = 'text';
apiInstance.stringOperationsPatternMatching(inputText, pattern, (error, data) => {
if (error) {
console.error(error);
} else {
console.log('Match found: ' + data.matchFound);
}
});
Replace YOUR_API_KEY
with your actual API key.
Conclusion
The Cloudmersive NLP API provides a wide range of functionalities to analyze and process natural language. In this article, we have walked through some examples of using the API with JavaScript. You can explore more functionalities of the API by referring to the official Cloudmersive NLP API documentation.