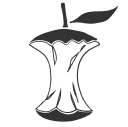
CORE
ScienceIntroduction to Core.ac.uk API
Core.ac.uk offers an API that allows developers to access a wide range of academic research articles. This service is especially useful for those who are developing research-based applications, academic tools, and bibliographic websites.
In this article, we will explore various examples of how you can use the Core.ac.uk API using JavaScript.
Getting Started
Firstly, we need to obtain an API key from Core.ac.uk API. After that, we can start querying the API. Let's start with a simple query to get a list of articles for a given search term.
const apiKey = 'InsertYourApiKeyHere'
const searchQuery = 'machine learning';
fetch(`https://api.core.ac.uk/v3/search/${searchQuery}?apiKey=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(data)
});
Here, we are using the fetch
function to send an HTTP request to the API with our API key and search query. The response from the API is returned in JSON format, which we can process as we wish.
Advanced Queries
We can also refine our search with other parameters like date range, category, file format, etc. Take a look at the following example which filters the articles for a given publication and specifies the number of results to return.
const publication = 'arXiv'
const maxResults = '10'
fetch(`https://api.core.ac.uk/v3/search/${publication}?apiKey=${apiKey}&pageSize=${maxResults}`)
.then(response => response.json())
.then(data => {
console.log(data)
});
Full Text Search
Another important feature of the Core.ac.uk API is its ability to search for full-text articles. Here is an example that finds articles mentioning "neural networks" in their full text.
const searchText = 'neural networks'
fetch(`https://api.core.ac.uk/v3/search/fulltext/${searchText}?apiKey=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(data)
});
We can also refine our search by specifying the fields to search in, like abstract, title, or full text.
Conclusion
In this article, we have explored various examples of using the Core.ac.uk API using JavaScript. With its advanced search capabilities and the wealth of academic articles available, Core.ac.uk API can be used to build amazing applications related to academic research.