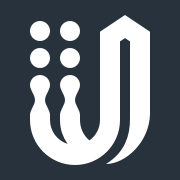
World Bank
ScienceExploring the World Bank API with JavaScript
The World Bank data API offers a rich source of information on global development indicators. In this article, we'll explore how to consume this API using JavaScript.
Getting Started
To start exploring the World Bank API, we need to obtain an API key. You can get one by registering on the World Bank's Data Hub.
Once you have an API key, you can start making requests to the API. The API uses the RESTful architecture and returns data in JSON format.
Example code
Fetching indicators
One of the main features of the World Bank API is the ability to retrieve indicators. Indicators are statistical measures that provide insights into development outcomes, such as poverty, education, or health.
Here's an example of how to fetch indicators using JavaScript:
const API_KEY = "YOUR_API_KEY";
const options = {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
};
fetch(`https://api.worldbank.org/v2/indicators?format=json&per_page=10&source=2&${API_KEY}`, options)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the Fetch API to make a GET request to the /indicators
endpoint of the World Bank API. The format
, per_page
, and source
parameters specify the output format, the number of results per page, and the data source (2 stands for world development indicators), respectively. The ${API_KEY}
part injects our API key into the URL.
Fetching countries
Another important feature of the World Bank API is the ability to retrieve country data. Here's an example of how to fetch countries:
const API_KEY = "YOUR_API_KEY";
const options = {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
};
fetch(`https://api.worldbank.org/v2/country?format=json&per_page=10&${API_KEY}`, options)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the same approach as before, but this time we're querying the /country
endpoint. The per_page
parameter specifies the number of results per page.
Fetching data for a specific country and indicator
Finally, let's see how to fetch data for a specific country and indicator. Here's an example:
const API_KEY = "YOUR_API_KEY";
const COUNTRY_CODE = "USA";
const INDICATOR_CODE = "NY.GDP.PCAP.CD";
const options = {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
};
fetch(`https://api.worldbank.org/v2/country/${COUNTRY_CODE}/indicator/${INDICATOR_CODE}?format=json&${API_KEY}`, options)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're querying the /country/{country_code}/indicator/{indicator_code}
endpoint, which returns data for a specific country and indicator. The COUNTRY_CODE
and INDICATOR_CODE
constants specify which country and indicator to retrieve.
Conclusion
In this article, we've explored how to use JavaScript to consume the World Bank data API. We've seen examples of how to fetch indicators, countries, and data for a specific country and indicator. Armed with this knowledge, you can start building your own applications that leverage the rich dataset provided by the World Bank API.