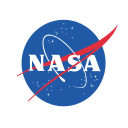
NASA
ScienceExploring the NASA Public API using JavaScript
The National Aeronautics and Space Administration (NASA) provides a plethora of data that is accessible through their public API. This API offers data on various aspects of space, astronomy, and earth science. In this blog, we will explore the API and learn to retrieve data using JavaScript.
Getting API Keys
To access the NASA API, we need to get an API key. We can obtain it by registering for a free account on https://api.nasa.gov/. Once we have an API key, we can use it in our requests to the API.
Making API Requests
We can make API requests to retrieve data by sending HTTP requests to the API endpoint. JavaScript provides several ways to retrieve data using http requests, including XMLHttpRequest, fetch(), and axios.
In this example, we will use the fetch() method to retrieve data from the API:
fetch(`https://api.nasa.gov/planetary/apod?api_key=${API_KEY}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code example, we use the fetch() method to make an HTTP GET request to the APOD (Astronomy Picture of the Day) endpoint of the NASA API, passing our API key as a query parameter. We then parse the response JSON data and log it to the console.
Example API Endpoints
Here are some examples of endpoints we can access with the NASA API.
APOD Images
The NASA API provides an endpoint to retrieve the Astronomy Picture of the Day (APOD) image and its information. Here's an example code to retrieve the image and its title using fetch() method in JavaScript:
fetch(`https://api.nasa.gov/planetary/apod?api_key=${API_KEY}`)
.then(response => response.json())
.then(data => {
const title = data['title'];
const imageUrl = data['url'];
console.log(`Title: ${title}`);
console.log(`Image URL: ${imageUrl}`);
})
.catch(error => console.error(error));
Mars Rover Photos
The NASA API provides an endpoint to retrieve images taken by the Mars rovers. Here's an example code to retrieve the 10 latest images taken by the Curiosity rover:
fetch(`https://api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos?sol=latest&api_key=${API_KEY}&page=1`)
.then(response => response.json())
.then(data => {
const images = data['photos'];
images.slice(0, 10).forEach(image => {
console.log(image['img_src']);
});
})
.catch(error => console.error(error));
Earth Observation Images
The NASA API also provides an endpoint to retrieve imagery of the earth. Here's an example code to retrieve a list of the latest imagery acquired by NASA's Earth Observation satellites:
fetch(`https://api.nasa.gov/planetary/earth/assets?lon=-149.99078798245626&lat=61.21887894558012&api_key=${API_KEY}`)
.then(response => response.json())
.then(data => {
const latestImage = data['date'];
console.log(`Latest Image: ${latestImage}`);
})
.catch(error => console.error(error));
Conclusion
The NASA API provides a vast collection of data for developers to explore. In this blog, we explored a few examples of data we can retrieve and displayed some sample code using fetch() method to retrieve this data using JavaScript.
To learn more about the NASA API and to explore more endpoints, visit their public API documentation: https://api.nasa.gov/.