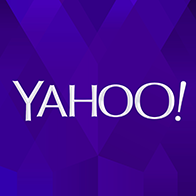
Yahoo! Weather
ScienceYahoo Weather API Documentation
Yahoo Weather API allows developers to access weather-related data for various locations. It returns weather forecasts for up to 10 days, as well as current weather data, sunrise and sunset times, humidity, wind speed, and more.
To use the Yahoo Weather API, you need to obtain an API key by registering on the Yahoo Developer Network. Once you have your API key, you can make API calls to retrieve the weather data.
API Endpoints
The Yahoo Weather API has a single RESTful endpoint that can be accessed via HTTP or HTTPS. The endpoint URL is:
https://weather-ydn-yql.media.yahoo.com/forecastrss
API Examples
Here are some examples of how you can use the Yahoo Weather API in JavaScript:
Example 1: Get Weather Data for a Specific Location
const apiURL = "https://weather-ydn-yql.media.yahoo.com/forecastrss";
const location = "Los Angeles, California";
const units = "f"; // specify units as f (Fahrenheit) or c (Celsius)
const apiID = "YOUR_API_ID"; // replace with your actual API ID
const xhr = new XMLHttpRequest();
xhr.open("GET", apiURL + `?location=${location}&format=json&u=${units}`);
xhr.setRequestHeader("Authorization", `Bearer ${apiID}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data); // display the weather data in the console
}
}
xhr.send();
Example 2: Get Sunrise and Sunset Times
const apiURL = "https://weather-ydn-yql.media.yahoo.com/forecastrss";
const location = "New York City";
const units = "f"; // specify units as f (Fahrenheit) or c (Celsius)
const apiID = "YOUR_API_ID"; // replace with your actual API ID
const xhr = new XMLHttpRequest();
xhr.open("GET", apiURL + `?location=${location}&format=json&u=${units}`);
xhr.setRequestHeader("Authorization", `Bearer ${apiID}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
const sunrise = data.current_observation.astronomy.sunrise;
const sunset = data.current_observation.astronomy.sunset;
console.log(`Sunrise: ${sunrise}, Sunset: ${sunset}`); // display the sunrise and sunset times in the console
}
}
xhr.send();
Example 3: Get Weather Data for Multiple Locations
const apiURL = "https://weather-ydn-yql.media.yahoo.com/forecastrss";
const locations = ["Seattle, Washington", "Chicago, Illinois", "Miami, Florida"];
const units = "f"; // specify units as f (Fahrenheit) or c (Celsius)
const apiID = "YOUR_API_ID"; // replace with your actual API ID
locations.forEach(location => {
const xhr = new XMLHttpRequest();
xhr.open("GET", apiURL + `?location=${location}&format=json&u=${units}`);
xhr.setRequestHeader("Authorization", `Bearer ${apiID}`);
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(`${location}: ${data.current_observation.condition.temperature}°F`);
}
}
xhr.send();
});
Conclusion
The Yahoo Weather API is a useful tool for developers who need to retrieve weather data for various locations. Through API calls, you can access weather forecasts for up to 10 days, as well as current weather data, sunrise and sunset times, humidity, wind speed, and more. By following the examples above, you can easily integrate the Yahoo Weather API into your JavaScript projects.