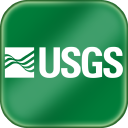
USGS Earthquake Hazards Program
ScienceExplore Earthquake Data with the USGS API
The United States Geological Survey (USGS) provides a public API to access information on earthquakes worldwide. This API allows developers to query earthquake data in real time using various parameters such as location, time, and magnitude.
Getting Started
To start exploring the earthquake data, we need to first make a request to the API endpoint. The endpoint for the USGS earthquake API is https://earthquake.usgs.gov/fdsnws/event/1/query.
Query Parameters
The following query parameters are available for filtering the results:
starttime
- start time of the earthquake (YYYY-MM-DD)endtime
- end time of the earthquake (YYYY-MM-DD)minmagnitude
- minimum magnitude of the earthquakemaxmagnitude
- maximum magnitude of the earthquakelatitude
- latitude of the locationlongitude
- longitude of the locationmaxradiuskm
- maximum radius around the locationorderby
- order of the results (time, magnitude, distance)
API Example Code in JavaScript
Below is an example on how to retrieve earthquake data with JavaScript.
const endpoint = "https://earthquake.usgs.gov/fdsnws/event/1/query";
// Set query parameters
const params = {
starttime: "2021-01-01",
endtime: "2021-02-01",
minmagnitude: 6.0,
latitude: 34.0522,
longitude: -118.2437,
maxradiuskm: 1000,
orderby: "time"
};
// Construct query string
const queryString = Object.keys(params)
.map(key => key + "=" + encodeURIComponent(params[key]))
.join("&");
// Make API request with fetch
fetch(endpoint + "?" + queryString)
.then(response => response.json())
.then(data => {
console.log(data);
});
This code retrieves all earthquakes with a magnitude of 6.0 or higher that occurred within a 1000 km radius of Los Angeles between January 1, 2021 and February 1, 2021. The resulting data is logged to the console.
Conclusion
With the USGS earthquake API, we can easily access and analyze earthquake data in real time using just a few lines of code. By applying different query parameters, we can hone in on specific information and gain a better understanding of seismology. The possibilities for exploration with this API is endless.