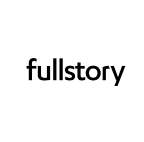
FullStory API
AnalyticsUsing the FullStory API with JavaScript
The FullStory API allows developers to programmatically access data from the FullStory platform, enabling customization and integration of FullStory's session replay and analytics features within your own applications.
Getting Started
To begin using the FullStory API with JavaScript, you'll need to have a FullStory account, generate an API key, and include the FullStory API client library in your project.
Generating an API Key
To generate an API key, log in to your FullStory account and navigate to Settings > Integrations > API Keys. Click the "Generate New API Key" button and copy the key to your clipboard.
Including the FullStory API Client Library
You can download the FullStory API client library from the FullStory Developer Docs. Once downloaded, include the library in your project by adding the following script tag to your HTML:
<script src="fullstory.min.js"></script>
Example API Requests
Once you have included the FullStory API client library in your project and have your API key, you're ready to start making API requests. Here are a few example API requests that you can use to get started.
Retrieve a Session Replay URL
To retrieve a session replay URL for a particular user and session ID, use the getReplayUrl
method:
FS.getReplayUrl({
user: "user@example.com",
sessionUrl: "https://app.fullstory.com/ui/ORG_ID/segment/SESSION_ID:PATH",
apiKey: "YOUR_API_KEY"
}, function(error, data) {
if (error) {
console.error(error);
} else {
console.log("Replay Url: " + data.replayUrl);
}
});
Search for Sessions
To search for sessions that match certain criteria, use the search
method:
FS.search({
apiKey: "YOUR_API_KEY",
query: "customerEmail:'user@example.com'",
limit: 10
}, function(error, data) {
if (error) {
console.error(error);
} else {
console.log(data);
}
});
Send Custom Events
To send custom events from your application to FullStory, use the event
method:
FS.event("addToCart", {
productId: "abc123",
price: 9.99,
quantity: 1
});
Conclusion
Using the FullStory API with JavaScript allows you to customize and integrate FullStory's session replay and analytics capabilities within your own applications. By following the examples provided in this guide, you can start making API requests to retrieve session replay URLs, search for sessions, and send custom events to FullStory.