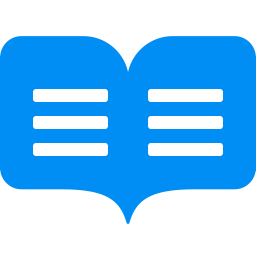
Woopra
AnalyticsExploring Woopra's Reporting API
Are you looking for a way to extract insight from your Woopra data to drive better business decisions? Woopra's Reporting API might just have everything you need. This flexible API exposes a range of data points and metrics that you can use to interrogate your customer behavior and gain valuable insight.
Getting started with Woopra's Reporting API
To get started, you will first need to obtain an API key. This can be done on your Woopra dashboard by navigating to Manage > API > Keys. From here, you can create a new key or use an existing one.
Once you have your API key, it's time to get familiar with the documentation. The Woopra Reporting API documentation can be found at https://docs.woopra.com/reference#the-reporting-api.
Example code in Javascript
Here are some sample codes you can use in Javascript to interact with Woopra's API:
Get a single visitor's details:
fetch('https://www.woopra.com/rest/2.4/reporting/profile', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY_HERE'
},
body: JSON.stringify({
'filters': {'operator': 'AND', 'values': [{'property': 'visitor', 'value': 'EMAIL_OR_COOKIE_ID_HERE'}]}
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
Get top referral traffic sources:
fetch('https://www.woopra.com/rest/2.4/reporting/profiles', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY_HERE'
},
body: JSON.stringify({
'filters': {'operator': 'AND', 'values': [{'property': 'event_name', 'value': 'pv'}, {'property': 'dimension', 'value': 'referrer'}]},
'grouping': {'operator': 'AND', 'values': [{'type': 'dimension', 'name': 'referrer'}]},
'metrics': [{'type': 'count', 'name': 'pv'}],
'ordering': [{'type': 'metric', 'name': 'pv', 'sort': 'descending'}],
'limit': 10
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
Get daily pageviews:
fetch('https://www.woopra.com/rest/2.4/reporting/profiles', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY_HERE'
},
body: JSON.stringify({
'filters': {'operator': 'AND', 'values': [{'property': 'event_name', 'value': 'pv'}]},
'grouping': {'operator': 'AND', 'values': [{'type': 'date', 'name': 'day', 'format': 'YYYY-MM-DD'}, {'type': 'dimension', 'name': 'hostname'}, {'type': 'dimension', 'name': 'path'}]},
'metrics': [{'type': 'count', 'name': 'pv'}],
'ordering': [{'type': 'date', 'name': 'day', 'sort': 'descending'}],
'limit': 10
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
Conclusion
With Woopra's Reporting API, you can easily extract data points and metrics that help you understand your customer behavior better. We hope the example codes above were helpful in getting you started. If you have any questions or need assistance, don't hesitate to reach out to the Woopra team. Happy coding!