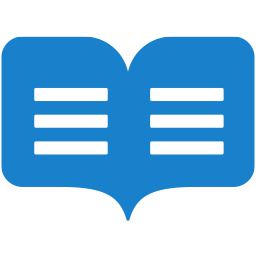
MixPanel
AnalyticsUsing Mixpanel API in JavaScript
Mixpanel API is a powerful tool used to collect, analyze and visualize user data for businesses. It allows developers to seamlessly integrate Mixpanel functionalities into their web application. In this article, we will explore some of the most commonly used APIs of Mixpanel in JavaScript.
Installation
To use Mixpanel APIs in your JavaScript project, you need to first include the Mixpanel library to your HTML file. You can do this by adding the following code to the head section of your HTML file.
<script type="text/javascript" src="//cdn.mxpnl.com/libs/mixpanel-2-latest.min.js"></script>
Initialization
To start using Mixpanel APIs in your application, you need to initialize Mixpanel with your project token. You can obtain the project token from your Mixpanel account settings.
var mixpanel = Mixpanel.init("YOUR_PROJECT_TOKEN");
Tracking Events
Mixpanel allows you to track events that occur in your web application to understand user behavior. The track
method is used to track events and can be called with the event name and any relevant properties associated with the event.
mixpanel.track('Click Button', {
'Button Name': 'Signup',
'Page Name': 'Homepage',
'User ID': '1234'
});
Setting User Properties
You can set user properties to further personalize your application experience for individual users. The people.set
method is used to set user properties. You can set any property that is relevant to your application.
mixpanel.people.set({
'$email': 'john.doe@gmail.com',
'Account Type': 'Pro',
'Subscription Plan': 'Weekly'
});
Creating User Profiles
Mixpanel also allows you to create user profiles for each individual user. User profiles store all data about a user, such as their behaviors, actions, and properties. The identify
method is called to create or update user profiles.
mixpanel.identify('USER_ID');
Tracking Revenue
Mixpanel also allows you to track revenue from your users. This data can help you understand how your users are spending money in your application. The people.trackCharge
method is used to track revenue.
mixpanel.people.trackCharge(10.99, {
'Shipping Method': 'Standard',
'Product ID': '1234'
});
These are just a few examples of the many APIs offered by Mixpanel. With Mixpanel's APIs in JavaScript, you can collect, analyze, and visualize user data to improve your application and user engagement.
If you want to explore more Mixpanel APIs, you can refer to the official documentation here.