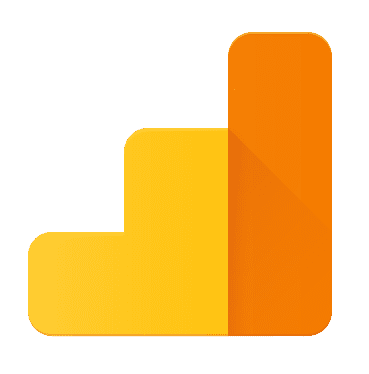
Google Analytics
AnalyticsHow to Use the Google Analytics API with NoCodeAPI
If you want to access your Google Analytics data programmatically, then NoCodeAPI's Google Analytics API is the perfect solution for you. This API makes it easy to pull data from Google Analytics into your application or website.
In this blog post, we will go over the steps to use the Google Analytics API with NoCodeAPI and provide some example code in JavaScript.
Setting Up
-
Start by visiting https://nocodeapi.com/google-analytics-api and clicking the "Connect" button to connect to your Google Analytics account.
-
Choose the website for which you want to use the API.
-
Once you've authorized the connection, copy the API endpoint URL and API key.
Example Code
To make API calls, you can use popular HTTP client libraries such as Axios or fetch. Below are some example code snippets in JavaScript using Axios:
Get Number of Sessions for Today
const axios = require('axios');
axios.get('https://api.nocodeapi.com/google-analytics/v1/sessions-today/:ga_id', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => {
console.log(response.data.sessions);
})
.catch(error => {
console.error(error);
});
Get Top 5 Pages for the Past Week
const axios = require('axios');
axios.get('https://api.nocodeapi.com/google-analytics/v1/top-page-views/:ga_id?days=7&limit=5', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => {
console.log(response.data.pages);
})
.catch(error => {
console.error(error);
});
Get User and Session Count for the Past Month
const axios = require('axios');
axios.get('https://api.nocodeapi.com/google-analytics/v1/user-and-session-count/:ga_id?monthly=true', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => {
console.log(response.data.users, response.data.sessions);
})
.catch(error => {
console.error(error);
});
Conclusion
With NoCodeAPI's Google Analytics API, it's easy to access your Google Analytics data programmatically. By using the example code provided in this blog post, you should be able to get started with the API in no time. Happy coding!